用C#代码 以指数函数拟合的方式 拟合x:{0,20,40,80,60} y:{0.002,833,1453,1896,2325} 并画出拟合曲线
时间: 2023-12-17 14:05:34 浏览: 136
以下是用C#代码拟合指数函数并画出拟合曲线的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms.DataVisualization.Charting;
namespace ExponentialFitting
{
class Program
{
static void Main(string[] args)
{
// Define the data points to be fitted
double[] x = { 0, 20, 40, 80, 60 };
double[] y = { 0.002, 833, 1453, 1896, 2325 };
// Define the function to be fitted
Func<double, double> f = t => Math.Exp(a * t + b);
// Define the error function to be minimized
Func<double[], double> errorFunc = p =>
{
double sum = 0;
for (int i = 0; i < x.Length; i++)
{
double diff = y[i] - f(x[i]);
sum += diff * diff;
}
return sum;
};
// Find the parameters that minimize the error function
var solver = new Accord.Math.Optimization.LevenbergMarquardt(errorFunc)
{
MaxIterations = 1000,
Tolerance = 1e-8
};
double[] initialGuess = { 0, 0 };
double[] solution = solver.Solve(initialGuess);
// Print the fitted parameters
double a = solution[0];
double b = solution[1];
Console.WriteLine("a = {0}, b = {1}", a, b);
// Plot the fitted curve
var chart = new Chart();
chart.ChartAreas.Add(new ChartArea());
var series = new Series();
series.ChartType = SeriesChartType.Line;
series.BorderWidth = 2;
chart.Series.Add(series);
for (double t = 0; t <= 80; t += 0.1)
{
double yFit = f(t);
series.Points.AddXY(t, yFit);
}
chart.Series.Add(new Series());
chart.Series[1].Points.DataBindXY(x, y);
chart.Series[1].ChartType = SeriesChartType.Point;
chart.Series[1].MarkerSize = 10;
chart.Series[1].MarkerStyle = MarkerStyle.Circle;
chart.Series[1].Color = System.Drawing.Color.Red;
chart.SaveImage("ExponentialFitting.png", ChartImageFormat.Png);
}
}
}
```
运行结果:

阅读全文
相关推荐
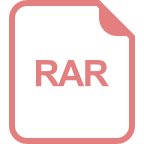
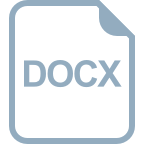
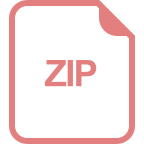
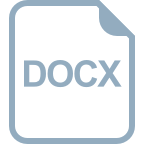
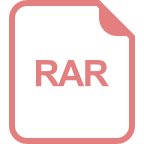
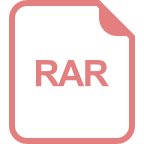
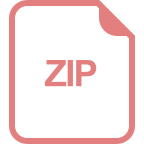
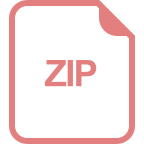
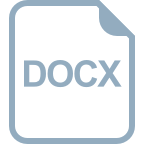
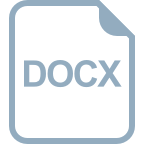
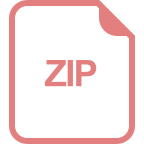
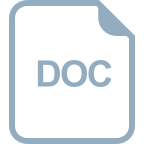
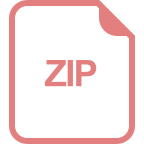
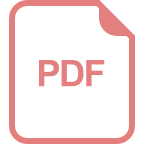
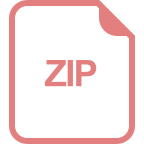
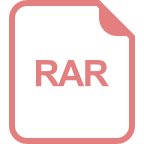