给出logistics的python代码
时间: 2023-11-12 11:12:09 浏览: 86
当然可以,以下是一个简单的logistics模型的Python代码:
```python
import numpy as np
def logistic(t, K, r, P0):
"""
Compute the logistic function for population growth. Takes as inputs:
t : array-like list or array of times
K : float, carrying capacity of population
r : float, population growth rate
P0 : int, initial population size
"""
exp_func = np.exp(r * t)
return K * P0 * exp_func / (K + P0 * (exp_func - 1))
# Example usage
t = np.linspace(0, 10, 100)
K = 10000
r = 0.1
P0 = 5000
population = logistic(t, K, r, P0)
```
相关问题
logistics回归python代码
以下是一个简单的logistic回归Python代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成随机数据
np.random.seed(0)
X = np.random.randn(100, 2)
y = np.random.randint(0, 2, 100)
# 定义sigmoid函数
def sigmoid(z):
return 1 / (1 + np.exp(-z))
# 定义损失函数
def loss(h, y):
return (-y * np.log(h) - (1 - y) * np.log(1 - h)).mean()
# 定义梯度下降函数
def gradient_descent(X, h, y):
return np.dot(X.T, (h - y)) / y.shape[0]
# 初始化参数
theta = np.zeros(X.shape[1])
# 设置学习率和迭代次数
learning_rate = 0.1
iterations = 1000
# 迭代更新参数
for i in range(iterations):
z = np.dot(X, theta)
h = sigmoid(z)
gradient = gradient_descent(X, h, y)
theta -= learning_rate * gradient
if i % 100 == 0:
print('loss:', loss(h, y))
# 绘制决策边界
x1_min, x1_max = X[:, 0].min() - 1, X[:, 0].max() + 1
x2_min, x2_max = X[:, 1].min() - 1, X[:, 1].max() + 1
xx1, xx2 = np.meshgrid(np.arange(x1_min, x1_max, 0.1),
np.arange(x2_min, x2_max, 0.1))
Z = sigmoid(np.dot(np.c_[xx1.ravel(), xx2.ravel()], theta))
Z = Z.reshape(xx1.shape)
plt.contourf(xx1, xx2, Z, alpha=0.4)
plt.scatter(X[:, 0], X[:, 1], c=y, alpha=0.8)
plt.show()
```
该代码使用随机生成的二维数据,通过logistic回归模型进行分类,并绘制出决策边界。
logistics回归模型如何用python代码实现
Logistic回归是一种用于分类问题的线性模型,它通过sigmoid函数将线性预测转换为概率形式。在Python中,我们可以使用scikit-learn库来实现逻辑回归模型。以下是简单的步骤:
```python
# 导入所需库
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
import pandas as pd
# 假设我们有一个名为data的DataFrame,其中包含特征(X)和目标变量(y)
data = pd.read_csv('your_data.csv') # 替换为实际数据文件路径
X = data.drop('target_column', axis=1) # 替换为目标列名
y = data['target_column']
# 数据预处理,如编码分类变量等
# ...
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 创建并初始化Logistic Regression模型
model = LogisticRegression()
# 训练模型
model.fit(X_train, y_train)
# 预测新样本
predictions = model.predict(X_test)
# 可视化结果或评估性能
```
在这个例子中,`train_test_split`用于划分数据集,`fit`方法用于拟合模型,`predict`方法用于做预测。为了提高模型性能,还可以尝试调整参数、正则化(如L1或L2惩罚)以及交叉验证。
阅读全文
相关推荐
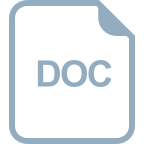
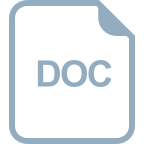
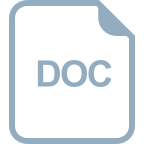
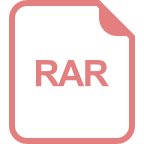











