C++类中如何使用pthread.h头文件在一个函数中给另一个带自定义参数的函数开启线程
时间: 2024-03-22 15:37:16 浏览: 61
要在一个函数中给另一个带自定义参数的函数开启线程,需要使用pthread_create函数。以下是示例代码:
```c++
#include <pthread.h>
// 定义线程参数结构体
struct thread_data {
int arg1;
char* arg2;
};
// 线程函数
void* thread_func(void* arg) {
struct thread_data* data = (struct thread_data*) arg;
int arg1 = data->arg1;
char* arg2 = data->arg2;
// do something with arg1 and arg2
pthread_exit(NULL);
}
// 主函数
int main() {
pthread_t thread;
struct thread_data data;
data.arg1 = 42;
data.arg2 = "hello";
// 创建线程
pthread_create(&thread, NULL, thread_func, (void*) &data);
// do something else
pthread_exit(NULL);
}
```
在主函数中,先定义了一个thread_data结构体,用于传递给线程函数的参数。然后通过pthread_create函数创建了一个新的线程,并把thread_data结构体的地址传递给了线程函数。在线程函数中,通过结构体指针获取传递进来的参数,并进行相应处理。注意,线程函数需要使用pthread_exit函数退出线程。
阅读全文
相关推荐
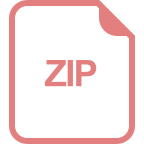
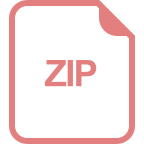



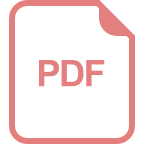
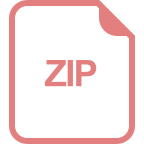
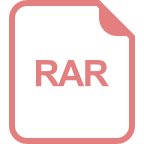
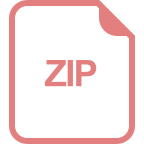
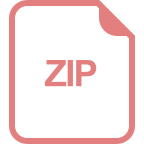
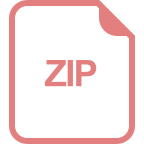





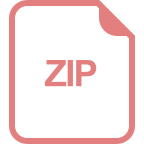
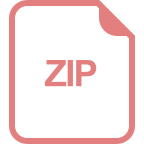