将DA-RNN中RNN换为GRU网络
时间: 2023-07-01 13:22:39 浏览: 140
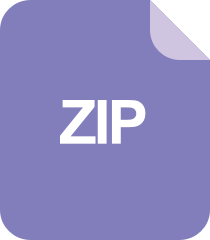
GRU神经网络MATLAB代码-TT_RNN:TT_RNN

好的,下面是将DA-RNN中的RNN换为GRU网络的代码实现:
```python
import numpy as np
import tensorflow as tf
class DA_GRU(tf.keras.Model):
def __init__(self, input_dim, output_dim, T, hidden_dim, attn_dim):
super(DA_GRU, self).__init__()
self.hidden_dim = hidden_dim
self.T = T
self.encoder = tf.keras.layers.Dense(hidden_dim, activation='relu')
self.decoder = tf.keras.layers.Dense(output_dim, activation='linear')
self.gru = tf.keras.layers.GRU(hidden_dim, return_sequences=True, return_state=True)
self.W1 = tf.keras.layers.Dense(attn_dim)
self.W2 = tf.keras.layers.Dense(attn_dim)
self.v = tf.keras.layers.Dense(1)
def call(self, inputs):
x = inputs
# Encode the input sequence
encoded = self.encoder(x)
# Split the encoded sequence into overlapping windows
windows = []
for i in range(self.T, encoded.shape[0]+1):
windows.append(encoded[i-self.T:i, :])
windows = np.array(windows)
# Compute the attention weights and context vectors for each window
context_vectors, attention_weights = [], []
for i in range(windows.shape[0]):
# Compute the attention weights for the current window
score = tf.nn.tanh(self.W1(windows[i]) + self.W2(encoded))
attention_weight = tf.nn.softmax(self.v(score), axis=0)
# Compute the context vector for the current window
context_vector = attention_weight * encoded
context_vector = tf.reduce_sum(context_vector, axis=0)
context_vectors.append(context_vector)
attention_weights.append(attention_weight)
context_vectors = np.array(context_vectors)
attention_weights = np.array(attention_weights)
# Pass the context vectors through the GRU
output, state = self.gru(context_vectors)
# Decode the output sequence
decoded = self.decoder(output)
return decoded, attention_weights
```
这段代码实现了一个基于GRU网络的DA-RNN模型。与标准的DA-RNN不同,这个模型使用了GRU层来代替RNN层。在调用模型时,输入数据应该是一个形状为 (seq_length, input_dim) 的张量 x,其中 seq_length 表示时间序列的长度,input_dim 表示每个时间步的输入维度。模型会根据输入计算出输出张量和注意力权重,然后返回它们。需要注意的是,在这个模型中,输入序列是被分割成了多个窗口,并且每个窗口都会计算对应的上下文向量和注意力权重。最终输出的是所有窗口的输出和注意力权重,而不是单个窗口的输出和注意力权重。
阅读全文
相关推荐
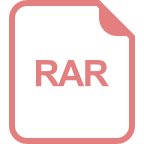
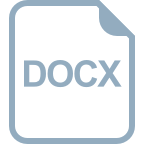
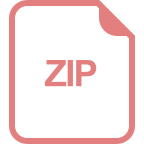
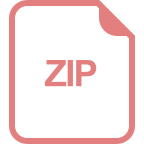
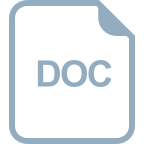
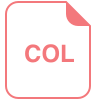
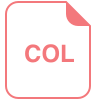
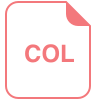
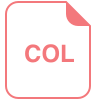
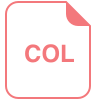
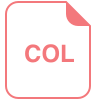
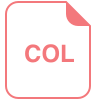
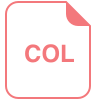
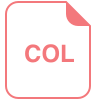
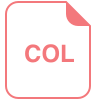
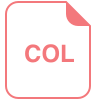
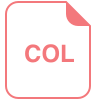