.net 6查询SQL
时间: 2023-11-22 15:57:01 浏览: 80
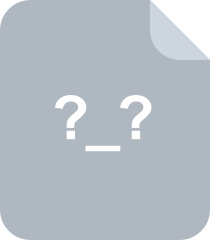
查询.sql
在 .NET 6 中,你可以使用 Entity Framework Core 或者 Dapper 来查询 SQL 数据库。
使用 Entity Framework Core 查询 SQL 数据库:
1. 安装 Entity Framework Core NuGet 包。
2. 在你的代码中创建 DbContext,并在 OnConfiguring 方法中指定数据库连接字符串。
```
public class MyDbContext : DbContext
{
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlServer("your_connection_string_here");
}
public DbSet<MyEntity> MyEntities { get; set; }
}
```
3. 定义实体类和 DbSet。
```
public class MyEntity
{
public int Id { get; set; }
public string Name { get; set; }
}
```
4. 使用 LINQ 查询数据。
```
using (var db = new MyDbContext())
{
var myEntity = db.MyEntities.FirstOrDefault(e => e.Id == 1);
}
```
使用 Dapper 查询 SQL 数据库:
1. 安装 Dapper NuGet 包。
2. 在你的代码中创建 IDbConnection,并指定数据库连接字符串。
```
var connection = new SqlConnection("your_connection_string_here");
```
3. 定义实体类和查询语句。
```
public class MyEntity
{
public int Id { get; set; }
public string Name { get; set; }
}
string sql = "SELECT * FROM MyEntities WHERE Id = @Id";
```
4. 使用 Query 方法查询数据。
```
var myEntity = connection.Query<MyEntity>(sql, new { Id = 1 }).FirstOrDefault();
```
阅读全文
相关推荐
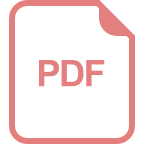
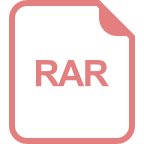
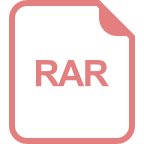
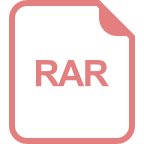
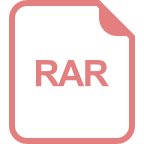
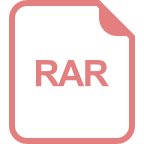
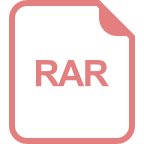
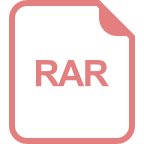
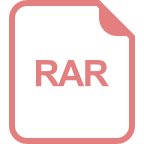
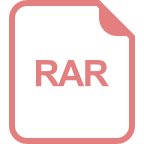
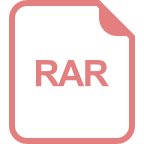
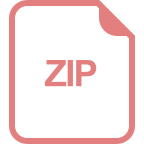
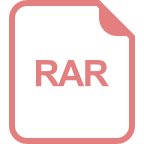