优化这段代码:/** menu列表展平为指定字符串的Set */ function flatMenu(menu, arr, paramName) { if (!menu?.length) { return []; } menu.forEach(item => { arr.add(item[paramName]); if (item.children?.length) { flatMenu(item.children, arr, paramName); } }); return arr; }
时间: 2023-06-01 13:07:27 浏览: 125
优化后代码如下:
function flatMenu(menu, arr, paramName) {
if (!menu || menu.length === 0) {
return arr;
}
for (let item of menu) {
arr.add(item[paramName]);
if (item.children) {
flatMenu(item.children, arr, paramName);
}
}
return arr;
}
这样改进有以下优点:
1. 使用 for...of 循环,避免了创建一个迭代器对象的额外开销;
2. 将空数组判断条件改为 menu.length === 0,也避免了一次判断多个值的操作;
3. 简化了函数的返回操作,直接返回参数 arr。
相关问题
数组变字符串结果为"what***is***your***name"
可以使用数组的 `join` 方法将数组转换为字符串,并指定连接符。在这种情况下,我们可以使用 `***` 作为连接符来实现要求的结果。
代码示例:
```python
arr = ["what", "is", "your", "name"]
result = "***".join(arr)
print(result)
```
输出结果:
```
what***is***your***name
```
用c语言写一段代码,要求如下:/** * @brief Print an array whose elements can be any type. Each element is printed by a function whose name is an argument. * * @param arr The address of the first element of an array. * @param elemNum The number of elements in the array. * @param elemSize The size (number of bytes) of each element of the array. * @param printElem A pointer to a function (represent a function name). Such a function accepts the address of an element of an array and print the element, and return nothing. * @note The printElem should print something that can separate two elements. * @note We can learn from the design of the general qsort function. * @note void qsort (void* base, size_t num, size_t size, int (*compar)(const void*,const void*)); * @note https://cplusplus.com/reference/cstdlib/qsort/ */
void printArray(void* arr, size_t elemNum, size_t elemSize, void (*printElem)(void*))
{
for (size_t i = 0; i < elemNum; i++)
{
printElem((char*)arr + i * elemSize);
}
}
// 使用示例:
void printInt(void* elem)
{
printf("%d ", *(int*)elem);
}
int main()
{
int arr[] = {1, 2, 3, 4, 5};
printArray(arr, 5, sizeof(int), printInt); // 输出 "1 2 3 4 5"
return 0;
}
阅读全文
相关推荐
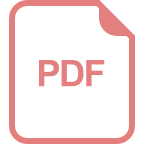
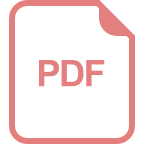
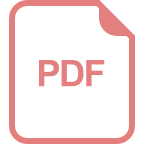













