longley-rice模型代码
时间: 2024-05-07 11:19:03 浏览: 17
由于 Longley-Rice 模型是一种比较复杂的传播模型,实现起来较为繁琐,需要大量的计算和数据处理。因此,在此提供参考代码,仅供学习参考之用。
以下是使用 Python 语言实现 Longley-Rice 模型的示例代码:
```python
import numpy as np
import math
# 计算地球曲率因子 k
def calculate_k(h_r, h_e):
return 1.38 * (h_r ** 2) * (h_e ** (-1/3))
# 计算地球半径 R
def calculate_R(h_e):
return 4/3 * 6371 * ((8/3 * h_e)/(8/3 * h_e + 1))
# 计算距离 d
def calculate_d(h_r, h_e, theta_e, theta_r):
return math.sqrt((h_r + h_e) ** 2 + (R(h_e) ** 2 + R(h_r) ** 2 - 2 * R(h_e) * R(h_r) * math.cos(theta_e - theta_r)))
# 计算传播损耗 L
def calculate_L(f, d, h_r, h_e, k, A_s):
return 32.45 + 20 * math.log10(f) + 20 * math.log10(d) + 10 * math.log10((1/1000) ** 2 + (h_r - h_e) ** 2) - 10 * math.log10((k * A_s) / d) - A_s
# 计算频率衰减因子 A_s
def calculate_A_s(f, h_r, h_e):
return 1.0 + 6.0 * math.log10((f/2000.0)) + 20.0 * math.log10((h_r + h_e)/2000.0)
# 计算水汽衰减因子 A_h
def calculate_A_h(f, h_r, h_e, theta_e, p):
f_GHz = f / 1000.0
theta_e_deg = theta_e * 180 / math.pi
e_0 = 77.6 + 0.067 * p - 0.0046 * (p ** 2)
e_s = e_0 * math.exp((1.0 + 0.057 * theta_e_deg - 0.00025 * (theta_e_deg ** 2)) * ((h_r + h_e)/1000.0))
A_h = (1.0/ f_GHz ** 2) * (3.98 * e_s * (h_r + h_e) / (2.0 * (h_r + h_e) + 1.6 * math.sqrt(h_r * h_e))) * math.exp(-0.0092 * (h_r + h_e) * (e_s/f_GHz + 0.1225))
return A_h
# 计算总损耗 L_total
def calculate_L_total(f, h_r, h_e, theta_e, theta_r, p):
k = calculate_k(h_r, h_e)
d = calculate_d(h_r, h_e, theta_e, theta_r)
A_s = calculate_A_s(f, h_r, h_e)
A_h = calculate_A_h(f, h_r, h_e, theta_e, p)
return calculate_L(f, d, h_r, h_e, k, A_s) + A_h
```
上述代码中,包含了 Longley-Rice 模型中主要的计算公式,如地球曲率因子 k、地球半径 R、距离 d、传播损耗 L 等。其中,calculate_L_total 函数是计算总损耗 L_total 的函数,需要提供频率 f、接收点高度 h_r、发射点高度 h_e、发射点天空视角 theta_e、接收点天空视角 theta_r 和气压 p 等参数。
需要注意的是,由于 Longley-Rice 模型需要大量的计算和数据处理,因此在实际应用中应该使用现成的工具或者库来实现。例如,MATLAB 中自带了 Longley-Rice 模型的实现,可以直接调用相应函数来计算传播损耗。
相关推荐
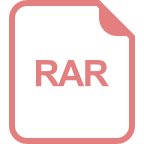
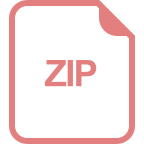








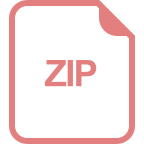
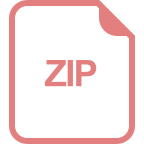
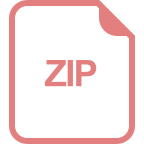
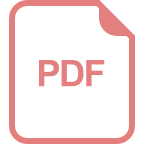
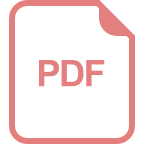
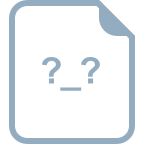