写一个bert-bilstm-crf算例
时间: 2023-08-28 19:07:51 浏览: 72
好的,下面是一个简单的BERT-BiLSTM-CRF代码示例:
首先,我们需要导入必要的库:
```
import torch
import torch.nn as nn
from transformers import BertModel
```
然后,我们定义一个BERT-BiLSTM-CRF类:
```
class BertBiLstmCrf(nn.Module):
def __init__(self, num_tags):
super(BertBiLstmCrf, self).__init__()
self.bert = BertModel.from_pretrained('bert-base-chinese')
self.dropout = nn.Dropout(0.1)
self.lstm = nn.LSTM(input_size=768, hidden_size=256, bidirectional=True, batch_first=True)
self.fc = nn.Linear(512, num_tags)
self.crf = CRF(num_tags, batch_first=True)
def forward(self, input_ids, token_type_ids=None, attention_mask=None, labels=None):
outputs = self.bert(input_ids, token_type_ids, attention_mask)
sequence_output = outputs[0]
sequence_output = self.dropout(sequence_output)
lstm_output, _ = self.lstm(sequence_output)
logits = self.fc(lstm_output)
if labels is not None:
loss = -self.crf(logits, labels, mask=attention_mask.byte(), reduction='mean')
return loss
else:
scores = self.crf.decode(logits, mask=attention_mask.byte())
return scores
```
在这个类中,我们首先使用BERT模型从Hugging Face Transformers库中导入预训练模型bert-base-chinese。然后,我们添加了一个dropout层和一个双向LSTM层。最后,我们使用一个线性分类层来预测每个标签的概率,并使用CRF层来解码序列标签。在forward()函数中,我们传递输入到BERT模型中,获取特征序列,然后将其传递给LSTM层。
如果传递了标签,则我们使用CRF层计算损失。否则,我们使用CRF层对序列标签进行解码,并返回预测的标签序列。
请注意,上面的代码示例省略了一些必要的库和变量定义。您需要使用您的特定库和数据集来适应代码。
希望这个简单的示例能够帮助您开始使用BERT-BiLSTM-CRF模型!
相关推荐
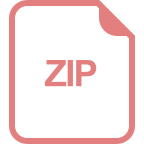
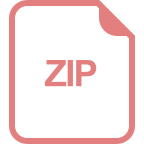
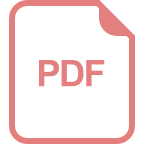














