jsoncpp如何返回当时时间,格式类似:20251101T163422102Z
时间: 2023-07-20 19:16:49 浏览: 75
要返回类似于`20251101T163422102Z`这样的时间格式,你可以使用C++标准库中的`strftime`函数将当前时间格式化为字符串,然后再将其转换为json格式。以下是一个示例代码:
```c++
#include <iostream>
#include <json/json.h>
#include <ctime>
int main() {
// 获取当前时间
std::time_t now = std::time(nullptr);
std::tm* local_now = std::gmtime(&now);
// 格式化时间字符串
char time_str[20];
std::strftime(time_str, sizeof(time_str), "%Y%m%dT%H%M%S%Z", local_now);
// 将时间转为json格式
Json::Value root(time_str);
// 输出json格式的时间
std::cout << root.toStyledString() << std::endl;
return 0;
}
```
这段代码会输出类似以下格式的json字符串:
```
"20211027T062727UTC"
```
你可以根据需要修改输出的时间格式,例如增加毫秒数等。
相关问题
jsoncpp如何返回当时的时间
jsoncpp本身并不提供获取当前时间的函数,但是可以通过C++标准库中的time和localtime函数获取当前时间,并将其转换为json格式。以下是一个示例代码:
```c++
#include <iostream>
#include <json/json.h>
#include <ctime>
int main() {
// 获取当前时间
std::time_t now = std::time(nullptr);
std::tm* local_now = std::localtime(&now);
// 将时间转为json格式
Json::Value root;
root["year"] = local_now->tm_year + 1900;
root["month"] = local_now->tm_mon + 1;
root["day"] = local_now->tm_mday;
root["hour"] = local_now->tm_hour;
root["minute"] = local_now->tm_min;
root["second"] = local_now->tm_sec;
// 输出json格式的时间
std::cout << root.toStyledString() << std::endl;
return 0;
}
```
这段代码会输出类似以下格式的json字符串:
```
{
"year" : 2021,
"month" : 10,
"day" : 26,
"hour" : 15,
"minute" : 30,
"second" : 0
}
```
你可以根据需要修改输出的时间格式。
使用jsoncpp把json路径读成json::value格式
抱歉,我之前的回答有误。`jsoncpp`库并不直接支持将JSON文件读取为`json::value`格式。但是,你可以使用`jsoncpp`库将JSON文件读取为`Json::Value`对象,然后根据需要进行转换。
以下是一个示例代码,演示如何使用`jsoncpp`库将JSON文件读取为`Json::Value`对象:
```cpp
#include <iostream>
#include <fstream>
#include <json/json.h>
int main() {
std::ifstream file("path/to/your/json/file.json");
if (!file.is_open()) {
std::cout << "Failed to open JSON file" << std::endl;
return 1;
}
// 创建Json::Value对象
Json::Value jsonValue;
// 使用Json::Reader解析JSON文件内容
Json::Reader reader;
if (!reader.parse(file, jsonValue)) {
std::cout << "Failed to parse JSON" << std::endl;
return 1;
}
// 打印JSON数据
std::cout << jsonValue << std::endl;
return 0;
}
```
请确保已经安装了`jsoncpp`库,并将其包含到你的项目中。上述代码将JSON文件内容解析为`Json::Value`对象,并可以通过`std::cout`打印出来。你可以根据自己的需要进一步处理或转换这个`Json::Value`对象。
相关推荐
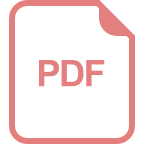
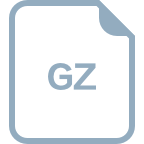
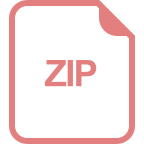












