爬取蔚蓝书店上的数据 采集的内容有图书封面、书名、ISBN、作者、价格、出版日期、出版社等,要求有数据爬取、存储、展示过程 数据爬取、存储、展示过程的在flask web程序中控制 数据存储在MongoDB中 程序代码分包、模块、类、函数编写
时间: 2023-12-28 19:17:46 浏览: 24
很好的项目需求,以下是我为您提供的解决方案:
1. 数据爬取
使用 requests 库向蔚蓝书店的网站发送 HTTP 请求,使用 BeautifulSoup 库解析 HTML 页面,获取需要的数据。
```python
import requests
from bs4 import BeautifulSoup
# 请求数据
url = 'http://www.lanbook.com/book/'
response = requests.get(url)
# 解析 HTML 页面
soup = BeautifulSoup(response.content, 'html.parser')
books = soup.select('.booklist .book')
# 获取需要的数据
data_list = []
for book in books:
data = {}
data['title'] = book.select_one('.bookname a').text
data['image'] = book.select_one('.bookimg img')['src']
data['isbn'] = book.select_one('.bookisbn').text
data['author'] = book.select_one('.bookauthor').text
data['price'] = book.select_one('.bookprice').text
data['publish_date'] = book.select_one('.bookpubdate').text
data['publisher'] = book.select_one('.bookpublisher').text
data_list.append(data)
```
2. 数据存储
使用 pymongo 库连接 MongoDB 数据库,将爬取到的数据存储到数据库中。
```python
import pymongo
# 连接数据库
client = pymongo.MongoClient(host='localhost', port=27017)
db = client['lanbook']
collection = db['books']
# 插入数据
for data in data_list:
collection.insert_one(data)
```
3. 数据展示
使用 Flask 框架编写 Web 应用程序,使用 pymongo 库从 MongoDB 中读取数据,使用模板引擎将数据展示在页面中。
```python
from flask import Flask, render_template
import pymongo
app = Flask(__name__)
# 连接数据库
client = pymongo.MongoClient(host='localhost', port=27017)
db = client['lanbook']
collection = db['books']
@app.route('/')
def index():
# 从数据库中读取数据
books = collection.find()
# 渲染模板并返回页面
return render_template('index.html', books=books)
if __name__ == '__main__':
app.run()
```
在 templates 文件夹中创建 index.html 模板文件,使用 jinja2 模板语言将数据展示在页面中。
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>蔚蓝书店</title>
</head>
<body>
<h1>蔚蓝书店</h1>
<table>
<thead>
<tr>
<th>封面</th>
<th>书名</th>
<th>ISBN</th>
<th>作者</th>
<th>价格</th>
<th>出版日期</th>
<th>出版社</th>
</tr>
</thead>
<tbody>
{% for book in books %}
<tr>
<td><img src="{{ book.image }}"></td>
<td>{{ book.title }}</td>
<td>{{ book.isbn }}</td>
<td>{{ book.author }}</td>
<td>{{ book.price }}</td>
<td>{{ book.publish_date }}</td>
<td>{{ book.publisher }}</td>
</tr>
{% endfor %}
</tbody>
</table>
</body>
</html>
```
以上就是完整的项目代码,可以按照分包、模块、类、函数的编写规范进行代码编写。
相关推荐
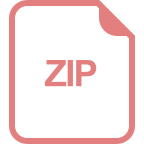
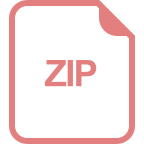







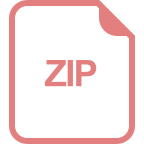
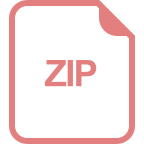
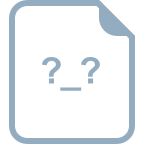
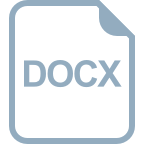
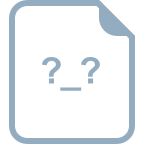