请给我编写一段程序,使用JavaFX利用对象和类,实现电视播放功能,至少有5个频道,电视没有打开的时候是黑屏显示。
时间: 2024-05-01 11:20:16 浏览: 23
以下是一个简单的JavaFX电视播放程序,实现了5个频道:
```java
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class TVPlayer extends Application {
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private Television television;
@Override
public void start(Stage primaryStage) throws Exception {
// 创建电视对象,初始频道为0
television = new Television();
// 创建播放区域,初始为黑屏
Rectangle screen = new Rectangle(WIDTH, HEIGHT, Color.BLACK);
StackPane screenPane = new StackPane(screen);
// 创建频道选择按钮
Button btnCh1 = new Button("频道1");
btnCh1.setOnAction(e -> television.setChannel(1));
Button btnCh2 = new Button("频道2");
btnCh2.setOnAction(e -> television.setChannel(2));
Button btnCh3 = new Button("频道3");
btnCh3.setOnAction(e -> television.setChannel(3));
Button btnCh4 = new Button("频道4");
btnCh4.setOnAction(e -> television.setChannel(4));
Button btnCh5 = new Button("频道5");
btnCh5.setOnAction(e -> television.setChannel(5));
HBox channelBox = new HBox(btnCh1, btnCh2, btnCh3, btnCh4, btnCh5);
channelBox.setAlignment(Pos.CENTER);
channelBox.setSpacing(10);
// 创建主布局
BorderPane root = new BorderPane();
root.setCenter(screenPane);
root.setBottom(channelBox);
// 监听频道变化,更新播放区域
television.addListener((observable, oldValue, newValue) -> {
screen.setFill(newValue.getColor());
});
// 显示窗口
Scene scene = new Scene(root, WIDTH, HEIGHT);
primaryStage.setScene(scene);
primaryStage.setTitle("电视播放器");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
class Television {
private int channel;
private Channel[] channels;
private Channel currentChannel;
private Listener listener;
public Television() {
channel = 0;
channels = new Channel[]{
new Channel(Color.RED),
new Channel(Color.GREEN),
new Channel(Color.BLUE),
new Channel(Color.YELLOW),
new Channel(Color.PURPLE)
};
currentChannel = channels[channel];
}
public void setChannel(int channel) {
this.channel = channel - 1;
currentChannel = channels[channel - 1];
if (listener != null) {
listener.onChange(currentChannel);
}
}
public void addListener(Listener listener) {
this.listener = listener;
}
interface Listener {
void onChange(Channel channel);
}
}
class Channel {
private Color color;
public Channel(Color color) {
this.color = color;
}
public Color getColor() {
return color;
}
}
```
该程序使用了JavaFX的布局和控件来实现界面,使用了对象和类来表示电视和频道,使用了接口和回调函数来实现频道变化时的更新。程序启动后,可以通过按钮选择不同的频道,播放区域会相应地更新。如果电视没有打开,播放区域会显示黑屏。
相关推荐
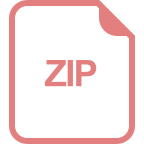
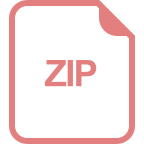














