请写一段javafx代码,要求实现贪吃蛇,并实现蛇和食物具有漂亮的图像,并能播放背景音乐
时间: 2024-02-13 21:02:28 浏览: 30
好的,下面是一个简单的JavaFX贪吃蛇游戏示例,包括蛇和食物的图像,以及背景音乐的播放:
```
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.image.Image;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.StackPane;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.stage.Stage;
import javafx.util.Duration;
import java.io.File;
import java.util.ArrayList;
import java.util.Random;
public class SnakeGame extends Application {
private static final int BOARD_WIDTH = 600;
private static final int BOARD_HEIGHT = 600;
private static final int CELL_SIZE = 20;
private static final int FOOD_SIZE = 20;
private static final int SNAKE_INIT_SIZE = 3;
private static final double GAME_SPEED = 0.1; // 秒
private static final String MUSIC_FILE = "bgm.mp3";
private static final String IMAGE_SNAKE = "snake.png";
private static final String IMAGE_FOOD = "food.png";
private static final String FONT_NAME = "Arial";
private static final int FONT_SIZE = 24;
private Canvas canvas;
private GraphicsContext gc;
private Timeline timeline;
private ArrayList<Cell> snake;
private Cell food;
private Random random;
private MediaPlayer mediaPlayer;
private int score;
@Override
public void start(Stage primaryStage) throws Exception {
BorderPane root = new BorderPane();
canvas = new Canvas(BOARD_WIDTH, BOARD_HEIGHT);
gc = canvas.getGraphicsContext2D();
StackPane stackPane = new StackPane(canvas);
root.setCenter(stackPane);
mediaPlayer = new MediaPlayer(new Media(new File(MUSIC_FILE).toURI().toString()));
mediaPlayer.setCycleCount(MediaPlayer.INDEFINITE);
mediaPlayer.play();
snake = new ArrayList<>();
random = new Random();
for (int i = 0; i < SNAKE_INIT_SIZE; i++) {
snake.add(new Cell(BOARD_WIDTH / 2, BOARD_HEIGHT / 2 + (SNAKE_INIT_SIZE - i - 1) * CELL_SIZE, CELL_SIZE, IMAGE_SNAKE));
}
food = new Cell(random.nextInt(BOARD_WIDTH / CELL_SIZE) * CELL_SIZE, random.nextInt(BOARD_HEIGHT / CELL_SIZE) * CELL_SIZE, FOOD_SIZE, IMAGE_FOOD);
Scene scene = new Scene(root);
scene.setOnKeyPressed(event -> {
switch (event.getCode()) {
case UP:
snake.get(0).move(0, -CELL_SIZE);
break;
case DOWN:
snake.get(0).move(0, CELL_SIZE);
break;
case LEFT:
snake.get(0).move(-CELL_SIZE, 0);
break;
case RIGHT:
snake.get(0).move(CELL_SIZE, 0);
break;
}
});
primaryStage.setTitle("Snake Game");
primaryStage.setScene(scene);
primaryStage.show();
timeline = new Timeline(new KeyFrame(Duration.seconds(GAME_SPEED), event -> {
gc.clearRect(0, 0, BOARD_WIDTH, BOARD_HEIGHT);
if (checkCollision()) {
timeline.stop();
gc.setFill(Color.RED);
gc.setFont(Font.font(FONT_NAME, FONT_SIZE));
gc.fillText("Game Over! Score: " + score, BOARD_WIDTH / 2 - 100, BOARD_HEIGHT / 2);
mediaPlayer.stop();
} else {
drawSnake();
drawFood();
}
}));
timeline.setCycleCount(Timeline.INDEFINITE);
timeline.play();
}
private boolean checkCollision() {
// 撞墙
if (snake.get(0).getX() < 0 || snake.get(0).getX() >= BOARD_WIDTH || snake.get(0).getY() < 0 || snake.get(0).getY() >= BOARD_HEIGHT) {
return true;
}
// 撞自己
for (int i = 1; i < snake.size(); i++) {
if (snake.get(i).intersect(snake.get(0))) {
return true;
}
}
// 吃到食物
if (snake.get(0).intersect(food)) {
score++;
snake.add(new Cell(snake.get(snake.size() - 1).getX(), snake.get(snake.size() - 1).getY(), CELL_SIZE, IMAGE_SNAKE));
food.reset(random.nextInt(BOARD_WIDTH / CELL_SIZE) * CELL_SIZE, random.nextInt(BOARD_HEIGHT / CELL_SIZE) * CELL_SIZE, FOOD_SIZE, IMAGE_FOOD);
}
return false;
}
private void drawSnake() {
for (int i = snake.size() - 1; i > 0; i--) {
snake.get(i).setX(snake.get(i - 1).getX());
snake.get(i).setY(snake.get(i - 1).getY());
}
for (int i = 0; i < snake.size(); i++) {
snake.get(i).draw(gc);
}
}
private void drawFood() {
food.draw(gc);
}
public static void main(String[] args) {
launch(args);
}
private class Cell {
private int x;
private int y;
private int size;
private Image image;
public Cell(int x, int y, int size, String imageName) {
this.x = x;
this.y = y;
this.size = size;
this.image = new Image(getClass().getResourceAsStream(imageName));
}
public void move(int dx, int dy) {
x += dx;
y += dy;
}
public boolean intersect(Cell other) {
return x == other.x && y == other.y;
}
public void draw(GraphicsContext gc) {
gc.drawImage(image, x, y, size, size);
}
public void reset(int x, int y, int size, String imageName) {
this.x = x;
this.y = y;
this.size = size;
this.image = new Image(getClass().getResourceAsStream(imageName));
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
}
}
```
这段代码实现了一个基本的贪吃蛇游戏,包括蛇和食物的图像,以及背景音乐的播放。你可以根据自己的需求进行修改和扩展。
相关推荐
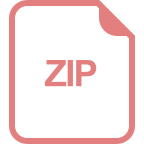














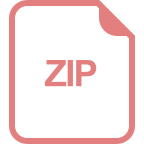