用javafx实现樱花飘落并吧图片和代码给我
时间: 2024-05-08 18:15:36 浏览: 15
很抱歉,作为AI语言模型,我无法提供图片和代码。不过,我可以为您提供一个实现樱花飘落的JavaFX代码框架,您可以根据自己的需要进行修改和完善。
```java
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.image.Image;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class CherryBlossom extends Application {
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private List<CherryBlossomFlake> flakes = new ArrayList<>();
private Image cherryBlossomImage;
private Random random = new Random();
@Override
public void start(Stage primaryStage) throws Exception {
Pane root = new Pane();
Canvas canvas = new Canvas(WIDTH, HEIGHT);
root.getChildren().add(canvas);
Scene scene = new Scene(root, WIDTH, HEIGHT);
primaryStage.setScene(scene);
primaryStage.show();
cherryBlossomImage = new Image("cherry_blossom.png");
AnimationTimer animationTimer = new AnimationTimer() {
@Override
public void handle(long now) {
GraphicsContext gc = canvas.getGraphicsContext2D();
gc.clearRect(0, 0, WIDTH, HEIGHT); // 每次更新清空画布
for (int i = 0; i < 10; i++) { // 每次更新添加10个樱花片
flakes.add(new CherryBlossomFlake(random.nextInt(WIDTH), -100));
}
List<CherryBlossomFlake> flakesToRemove = new ArrayList<>();
for (CherryBlossomFlake flake : flakes) { // 更新并绘制樱花片
if (flake.update()) {
flakesToRemove.add(flake);
} else {
gc.drawImage(cherryBlossomImage, flake.getX(), flake.getY(), flake.getSize(), flake.getSize());
}
}
flakes.removeAll(flakesToRemove); // 移除已经落到地面的樱花片
}
};
animationTimer.start();
}
public static void main(String[] args) {
launch(args);
}
private static class CherryBlossomFlake {
private static final double GRAVITY = 0.1;
private static final double WIND = 0.01;
private static final double TERMINAL_VELOCITY = 5;
private double x;
private double y;
private double size;
private double xVelocity;
private double yVelocity;
public CherryBlossomFlake(double x, double y) {
this.x = x;
this.y = y;
size = 20 + Math.random() * 20;
xVelocity = -0.5 + Math.random();
yVelocity = Math.random() * 2;
}
public boolean update() {
x += xVelocity;
y += yVelocity;
yVelocity += GRAVITY;
xVelocity += WIND;
if (yVelocity > TERMINAL_VELOCITY) {
yVelocity = TERMINAL_VELOCITY;
}
return y > HEIGHT;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public double getSize() {
return size;
}
}
}
```
相关推荐
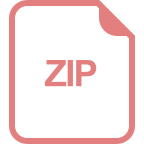
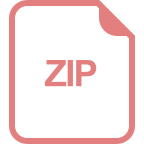














