在基于图像迭代的图像风格迁移算法中加入总变差损失
时间: 2023-11-06 14:07:02 浏览: 99
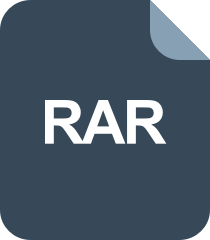
基于VGG的图像风格迁移算法实现
好的,基于图像迭代的图像风格迁移算法中加入总变差损失的方法如下:
我们以 Gatys 等人提出的基于图像迭代的图像风格迁移算法为例。该算法的核心思想是利用一个预训练的卷积神经网络来提取图像的特征,然后通过最小化输入图像和风格图像的特征的距离来实现图像风格的迁移。其损失函数通常由三部分组成:内容损失、风格损失和总变差损失。
总变差损失的作用是使得生成的图像更加平滑,避免出现过多的噪点和细节。在算法中,我们可以将总变差损失加入到损失函数中,以平衡内容损失和风格损失。
以下是基于 PyTorch 实现的例子代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision.transforms as transforms
import torchvision.models as models
from PIL import Image
# 定义图像预处理函数
def image_loader(image_name, imsize):
loader = transforms.Compose([
transforms.Resize(imsize), # 调整图像大小
transforms.CenterCrop(imsize), # 裁剪图像中心部分
transforms.ToTensor()]) # 将图像转换为张量
image = Image.open(image_name)
image = loader(image).unsqueeze(0)
return image.to(torch.float)
# 定义内容损失函数
class ContentLoss(nn.Module):
def __init__(self, target):
super(ContentLoss, self).__init__()
self.target = target.detach()
def forward(self, input):
self.loss = F.mse_loss(input, self.target)
return input
# 定义风格损失函数
class StyleLoss(nn.Module):
def __init__(self, target_feature):
super(StyleLoss, self).__init__()
self.target = gram_matrix(target_feature).detach()
def forward(self, input):
G = gram_matrix(input)
self.loss = F.mse_loss(G, self.target)
return input
# 定义总变差损失函数
def TotalVariationLoss(x):
h, w = x.shape[-2:]
return torch.sum(torch.abs(x[:, :, :, :-1] - x[:, :, :, 1:])) + \
torch.sum(torch.abs(x[:, :, :-1, :] - x[:, :, 1:, :]))
# 定义 VGG19 神经网络
class VGGNet(nn.Module):
def __init__(self):
super(VGGNet, self).__init__()
self.select = ['0', '5', '10', '19', '28']
self.vgg19 = models.vgg19(pretrained=True).features
def forward(self, x):
features = []
for name, layer in self.vgg19._modules.items():
x = layer(x)
if name in self.select:
features.append(x)
return features
# 定义 gram 矩阵函数
def gram_matrix(input):
a, b, c, d = input.size()
features = input.view(a * b, c * d)
G = torch.mm(features, features.t())
return G.div(a * b * c * d)
# 定义图像风格迁移函数
def stylize(content_image, style_image, num_steps, style_weight, content_weight, tv_weight):
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
imsize = 512 if torch.cuda.is_available() else 256
# 加载图像
content = image_loader(content_image, imsize).to(device)
style = image_loader(style_image, imsize).to(device)
# 定义模型和目标特征
vgg = VGGNet().to(device).eval()
content_features = vgg(content)
style_features = vgg(style)
# 初始化目标图像
target = content.clone().requires_grad_(True).to(device)
# 定义损失函数
content_loss, style_loss, tv_loss = 0, 0, 0
criterion = nn.MSELoss()
optimizer = optim.LBFGS([target])
# 迭代优化目标图像
for i in range(num_steps):
def closure():
nonlocal content_loss, style_loss, tv_loss
optimizer.zero_grad()
target_features = vgg(target)
# 计算内容损失
content_loss = criterion(target_features[1], content_features[1])
for t in range(2, len(content_features)):
content_loss += criterion(target_features[t], content_features[t])
# 计算风格损失
style_loss = 0
for t in range(len(style_features)):
style_loss += StyleLoss(target_features[t]).loss
style_loss *= style_weight
# 计算总变差损失
tv_loss = TotalVariationLoss(target)
tv_loss *= tv_weight
# 计算总损失
loss = content_weight * content_loss + style_loss + tv_loss
loss.backward()
return loss
optimizer.step(closure)
return target
```
在上面的代码中,我们定义了 `TotalVariationLoss` 函数来计算总变差损失,然后在 `stylize` 函数中,将总变差损失乘以一个超参数加入到原来的损失函数中,以平衡内容损失和风格损失。最后,我们使用 L-BFGS 优化器来迭代优化目标图像。
阅读全文
相关推荐
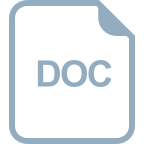
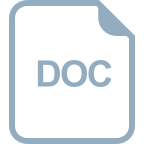


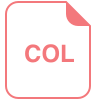
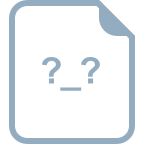
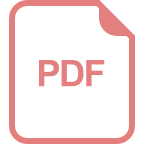
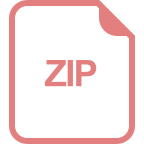
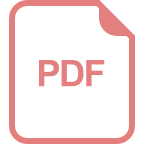
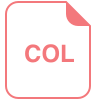
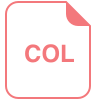
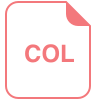
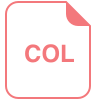
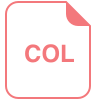
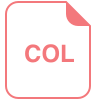
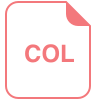
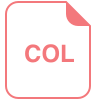