optimizer = tf.train.AdamOptimizer(0.001) train = optimizer.minimize(loss)
时间: 2024-05-20 14:19:37 浏览: 17
This code defines an optimizer using the Adam algorithm with a learning rate of 0.001. It then defines a training operation that minimizes the loss function using this optimizer. The optimizer adjusts the model parameters to minimize the difference between the predicted output and the actual output. The training operation updates the model parameters based on the gradients of the loss function with respect to the parameters. This code is commonly used in machine learning and deep learning applications to train neural networks.
相关问题
tf.train.AdamOptimizer
`tf.train.AdamOptimizer` 是 TensorFlow 中的一个优化器,用于优化神经网络的参数以最小化损失函数。
Adam 代表自适应矩估计优化器(Adaptive Moment Estimation Optimizer),是一种基于梯度下降算法的优化器。与传统的梯度下降优化器相比,Adam 优化器具有更快的收敛速度和更好的性能。
`tf.train.AdamOptimizer` 的使用方法如下:
```python
optimizer = tf.train.AdamOptimizer(learning_rate=0.001)
train_op = optimizer.minimize(loss)
```
其中,`learning_rate` 表示学习率,即每次迭代中调整参数的程度,`loss` 是损失函数。在训练过程中,我们可以反复执行 `train_op` 操作来更新模型的参数,使得损失函数逐渐减小,最终得到一个优秀的模型。
import tensorflow as tf # 定义输入的占位符 x = tf.placeholder(tf.float32, [None, 1280, 1024, 3]) y = tf.placeholder(tf.float32, [None, num_classes]) # num_classes表示类别数目 # 定义卷积层 conv1 = tf.layers.conv2d(inputs=x, filters=32, kernel_size=[3, 3], padding="same", activation=tf.nn.relu) pool1 = tf.layers.max_pooling2d(inputs=conv1, pool_size=[2, 2], strides=2) conv2 = tf.layers.conv2d(inputs=pool1, filters=64, kernel_size=[3, 3], padding="same", activation=tf.nn.relu) pool2 = tf.layers.max_pooling2d(inputs=conv2, pool_size=[2, 2], strides=2) # 定义全连接层 flatten = tf.reshape(pool2, [-1, 320 * 256 * 64]) fc1 = tf.layers.dense(inputs=flatten, units=256, activation=tf.nn.relu) dropout1 = tf.layers.dropout(inputs=fc1, rate=0.4) fc2 = tf.layers.dense(inputs=dropout1, units=num_classes) # 定义损失函数和优化器 loss = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=y, logits=fc2)) optimizer = tf.train.AdamOptimizer(learning_rate=0.001).minimize(loss) # 定义评估指标 correct_prediction = tf.equal(tf.argmax(fc2, 1), tf.argmax(y, 1)) accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
这段代码定义了一个基本的卷积神经网络模型,包括输入、卷积层、全连接层、损失函数和评估指标。具体来说:
- 输入占位符:x 表示输入的图片,y 表示图片对应的标签,num_classes 表示类别数目,因为是多分类问题,所以 y 是一个大小为 [None, num_classes] 的矩阵,其中 None 表示数据集大小可以是任意值。
- 卷积层:使用了两个卷积层和池化层来提取图片的特征。第一个卷积层有 32 个滤波器,大小为 3x3,使用 ReLU 激活函数,输出大小不变,使用 same 填充方式。第一个池化层使用 2x2 的窗口和步长,将输出大小减半。第二个卷积层有 64 个滤波器,大小为 3x3,使用 ReLU 激活函数,输出大小不变,使用 same 填充方式。第二个池化层使用 2x2 的窗口和步长,将输出大小减半。
- 全连接层:使用一个全连接层将卷积层的输出映射到 num_classes 个类别上。首先使用 flatten 函数将池化层的输出展平为一个一维向量,然后使用一个大小为 256 的隐藏层,使用 ReLU 激活函数,使用 dropout 技术来防止过拟合。
- 损失函数和优化器:使用 softmax_cross_entropy_with_logits 函数作为损失函数,AdamOptimizer 作为优化器,学习率为 0.001。
- 评估指标:使用 equal 函数来计算预测结果和真实标签是否一致,将结果转换为浮点数,然后使用 reduce_mean 函数计算平均值,得到准确率。
相关推荐
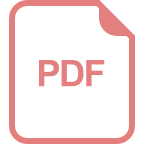













