springboot监听系统执行过的sql语句并存入sql执行历史表中
时间: 2023-06-25 22:04:24 浏览: 49
可以使用Spring Boot提供的AOP(面向切面编程)功能实现此功能。具体步骤如下:
1. 创建一个`SqlExecutionListener`类,实现`ApplicationListener`接口,监听`ApplicationReadyEvent`事件,在应用启动时初始化SQL执行历史表。
```
@Component
public class SqlExecutionListener implements ApplicationListener<ApplicationReadyEvent> {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public void onApplicationEvent(ApplicationReadyEvent applicationReadyEvent) {
// 初始化SQL执行历史表
jdbcTemplate.execute("CREATE TABLE IF NOT EXISTS sql_history (id INT PRIMARY KEY AUTO_INCREMENT, sql_text TEXT, create_time DATETIME DEFAULT CURRENT_TIMESTAMP)");
}
}
```
2. 创建一个`SqlExecutionAspect`类,使用`@Aspect`注解声明切面,在切面中定义一个`@Around`注解的方法,用来拦截所有DAO层的SQL执行,将SQL保存到SQL执行历史表中。
```
@Aspect
@Component
public class SqlExecutionAspect {
@Autowired
private JdbcTemplate jdbcTemplate;
@Around("execution(* com.example.dao..*(..))")
public Object logSql(ProceedingJoinPoint joinPoint) throws Throwable {
String sql = joinPoint.getArgs()[0].toString(); // 获取DAO层执行的SQL语句
Object result = joinPoint.proceed(); // 执行DAO层方法
// 将SQL保存到SQL执行历史表中
jdbcTemplate.update("INSERT INTO sql_history (sql_text) VALUES (?)", sql);
return result;
}
}
```
3. 在`application.properties`配置文件中添加数据源相关的配置。
```
spring.datasource.url=jdbc:mysql://localhost:3306/test
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
```
通过以上步骤,应用启动后,所有DAO层执行的SQL语句都会被保存到SQL执行历史表中。
相关推荐
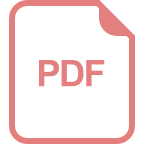
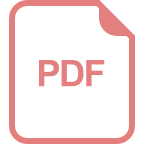














