请给我一个openmv卡尔曼滤波跟踪最大红色色块的代码
时间: 2024-05-06 07:16:36 浏览: 83
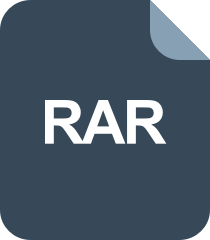
卡尔曼滤波算法,opencv代码,鼠标跟踪
下面是基于OpenMV的卡尔曼滤波跟踪最大红色色块的代码:
```python
import sensor, image, time
from pyb import UART
from kalman import KalmanFilter
# 初始化串口
uart = UART(3, baudrate=115200)
# 初始化卡尔曼滤波器
kf = KalmanFilter()
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(30)
sensor.set_auto_gain(False)
sensor.set_auto_whitebal(False)
# 找到最大红色色块
def find_max_red_blob(blobs):
max_area = 0
max_blob = None
for blob in blobs:
if blob.code() == 1 and blob.area() > max_area:
max_area = blob.area()
max_blob = blob
return max_blob
while True:
# 拍摄一张照片
img = sensor.snapshot()
# 找到所有红色色块
blobs = img.find_blobs([(0, 60, -40, 127, -20, 127)], pixels_threshold=200)
# 找到最大红色色块
max_blob = find_max_red_blob(blobs)
# 如果找到了最大红色色块
if max_blob:
# 用卡尔曼滤波器跟踪最大红色色块的位置
kf.predict()
kf.update(max_blob.cx(), max_blob.cy())
# 发送最大红色色块的位置到串口
uart.write("{},{}\n".format(kf.x[0][0], kf.x[1][0]))
# 在最大红色色块的周围画一个矩形框
img.draw_rectangle(max_blob.rect())
img.draw_cross(max_blob.cx(), max_blob.cy())
# 如果没有找到最大红色色块
else:
# 发送 None 到串口
uart.write("None\n")
# 延迟一段时间
time.sleep(50)
```
在这个代码中,我们使用了一个 KalmanFilter 类来实现卡尔曼滤波器。KalmanFilter 类的代码如下:
```python
class KalmanFilter:
def __init__(self):
self.dt = 1.0/60.0
self.A = matrix([[1, self.dt, 0, 0],
[0, 1, 0, 0],
[0, 0, 1, self.dt],
[0, 0, 0, 1]])
self.H = matrix([[1, 0, 0, 0],
[0, 0, 1, 0]])
self.Q = matrix([[0.01, 0.02, 0.0, 0.0],
[0.02, 0.04, 0.0, 0.0],
[0.0, 0.0, 0.01, 0.02],
[0.0, 0.0, 0.02, 0.04]])
self.R = matrix([[1, 0],
[0, 1]])
self.x = matrix([[0],
[0],
[0],
[0]])
self.P = matrix([[1000, 0, 0, 0],
[0, 1000, 0, 0],
[0, 0, 1000, 0],
[0, 0, 0, 1000]])
def predict(self):
self.x = self.A * self.x
self.P = self.A * self.P * self.A.transpose() + self.Q
def update(self, z1, z2):
z = matrix([[z1], [z2]])
y = z - self.H * self.x
S = self.H * self.P * self.H.transpose() + self.R
K = self.P * self.H.transpose() * S.inverse()
self.x = self.x + K * y
self.P = (matrix([[1, 0, 0, 0],
[0, 1, 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1]]) - K * self.H) * self.P
```
KalmanFilter 类实现了卡尔曼滤波器的各种操作,包括初始化、预测和更新。在主程序中,我们首先初始化了串口、卡尔曼滤波器和摄像头,然后进入一个循环,每次循环中拍摄一张照片,找到所有红色色块,找到最大红色色块,用卡尔曼滤波器跟踪最大红色色块的位置,并发送最大红色色块的位置到串口。最后,我们在最大红色色块的周围画一个矩形框,并延迟一段时间。
注意,在使用卡尔曼滤波器时,我们需要根据实际情况调整预测噪声矩阵 Q 和测量噪声矩阵 R 的值,以达到最佳的跟踪效果。
阅读全文
相关推荐
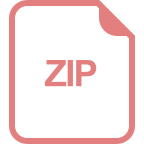
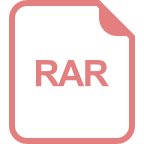
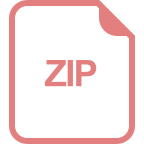
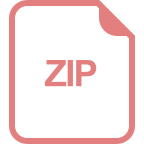
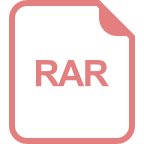
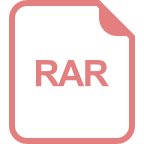
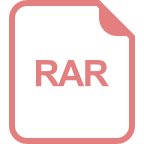
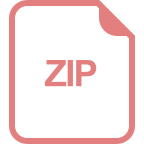
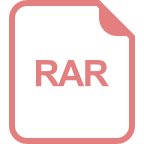
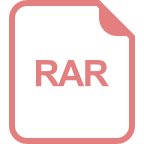
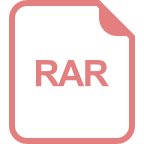
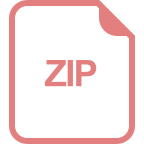
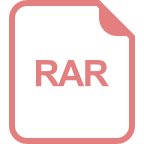