golang http server 代码
时间: 2023-09-12 22:02:52 浏览: 159
以下是一个简单的 Golang HTTP 服务器代码示例:
```go
package main
import (
"fmt"
"net/http"
)
func main() {
// 定义路由处理函数
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, world!")
})
// 启动 HTTP 服务器
if err := http.ListenAndServe(":8080", nil); err != nil {
fmt.Println(err)
}
}
```
该示例中,我们首先通过 `http.HandleFunc` 函数定义了一个路由处理函数,用于处理所有的 HTTP 请求。在本例中,我们只是简单地向客户端发送了一条消息。
接下来,我们调用 `http.ListenAndServe` 函数启动了一个 HTTP 服务器,监听在 8080 端口上。如果有任何错误发生,我们会将其打印出来。
相关问题
使用golang编写server
使用Golang编写一个简单的HTTP服务器可以有多种方式。下面是两种常见的实现方式:
第一种方式是使用`http.HandleFunc`函数和一个处理函数来实现。代码如下所示:
```go
package main
import (
"fmt"
"net/http"
)
func indexHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "hello world")
}
func main() {
http.HandleFunc("/", indexHandler)
http.ListenAndServe(":8000", nil)
}
```
在这个例子中,我们定义了一个`indexHandler`函数来处理根路径的请求。当有请求到达时,`indexHandler`函数会将"hello world"作为响应写入到`http.ResponseWriter`中。
第二种方式是使用自定义的处理器类型和`http.Handle`函数来实现。代码如下所示:
```go
package main
import (
"fmt"
"net/http"
)
type indexHandler struct {
content string
}
func (ih *indexHandler) ServeHTTP(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, ih.content)
}
func main() {
http.Handle("/", &indexHandler{content: "hello world!"})
http.ListenAndServe(":8001", nil)
}
```
在这个例子中,我们定义了一个`indexHandler`类型,并实现了`ServeHTTP`方法。当有请求到达时,`ServeHTTP`方法会将`indexHandler`的`content`字段作为响应写入到`http.ResponseWriter`中。
无论是哪种方式,我们都需要使用`http.ListenAndServe`函数来启动服务器。这个函数会监听指定的地址,并将请求交给指定的处理器来处理。
希望这个例子能帮助你理解如何使用Golang编写一个简单的HTTP服务器。
#### 引用[.reference_title]
- *1* *2* *3* [golang http Server介绍](https://blog.csdn.net/zrg3699/article/details/122280399)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
golang分布式缓存代码
以下是一个简单的基于Golang的分布式缓存代码。
```go
package main
import (
"fmt"
"net"
"net/http"
"net/rpc"
"time"
)
type Cache struct {
data map[string]string
}
func (c *Cache) Get(key string, value *string) error {
if val, ok := c.data[key]; ok {
*value = val
return nil
}
return fmt.Errorf("Key not found: %s", key)
}
func (c *Cache) Set(kv [2]string, reply *bool) error {
c.data[kv[0]] = kv[1]
*reply = true
return nil
}
func main() {
cache := &Cache{data: make(map[string]string)}
rpc.Register(cache)
rpc.HandleHTTP()
listener, err := net.Listen("tcp", ":1234")
if err != nil {
panic(err)
}
go http.Serve(listener, nil)
fmt.Println("Server started...")
for {
time.Sleep(time.Second)
}
}
```
这个代码实现了两个RPC方法:Get和Set。Get方法用于获取缓存中的值,Set方法用于设置缓存的值。
在main函数中,我们使用net包创建一个TCP监听器,然后启动一个Go协程来处理HTTP请求。我们使用RPC包注册缓存对象,并通过HTTP处理程序处理所有RPC请求。
这个代码只是一个简单的示例,真实的分布式缓存系统需要考虑更多的问题,例如数据一致性、节点故障处理等等。
阅读全文
相关推荐
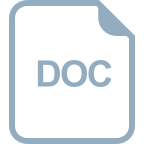
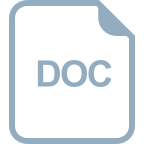
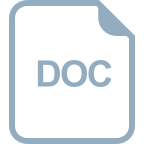
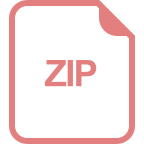
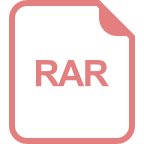
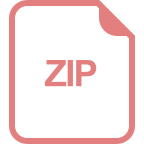
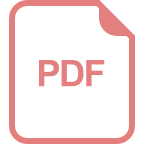
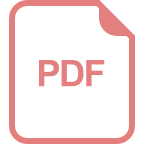
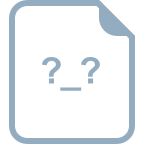
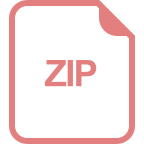
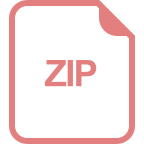
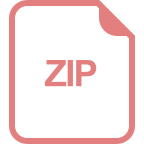
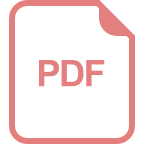
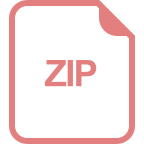
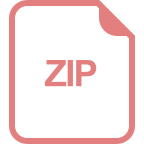

