用c语言写一个能处理3个字符串,按由小到大的顺序输出,用swap函数和时针写的代码
时间: 2024-02-29 14:53:05 浏览: 65
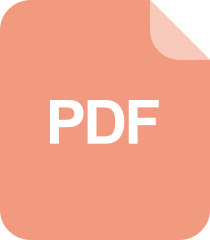
C语言实现3个数从小到大排序/输出的方法示例
以下是用C语言编写的能处理3个字符串并按由小到大排序输出的代码,使用了 `swap` 函数和时钟函数:
```c
#include <stdio.h>
#include <string.h>
#include <time.h>
void swap(char *s1, char *s2) {
char temp[100];
strcpy(temp, s1);
strcpy(s1, s2);
strcpy(s2, temp);
}
int main() {
char str1[100], str2[100], str3[100];
printf("请输入三个字符串:\n");
scanf("%s%s%s", str1, str2, str3);
// 按由小到大排序
clock_t start = clock();
if (strcmp(str1, str2) > 0) swap(str1, str2);
if (strcmp(str1, str3) > 0) swap(str1, str3);
if (strcmp(str2, str3) > 0) swap(str2, str3);
clock_t end = clock();
printf("排序后的字符串为:\n");
printf("%s\n%s\n%s\n", str1, str2, str3);
printf("排序用时:%f秒", (double)(end - start) / CLOCKS_PER_SEC);
return 0;
}
```
在上面的代码中,我们首先使用 `scanf` 函数从用户输入中读取三个字符串。然后,我们使用 `strcmp` 函数比较字符串,如果需要交换两个字符串的位置,则调用 `swap` 函数。最后,我们使用 `printf` 函数输出排序后的字符串,并使用 `clock` 函数计算排序所需的时间。
阅读全文
相关推荐















