Java gui生成一个简易记事本
时间: 2024-05-12 18:13:42 浏览: 105
好的,以下是一个简易的记事本GUI的Java代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class SimpleNotepad extends JFrame implements ActionListener {
private JTextArea textArea = new JTextArea();
private JMenuBar menuBar = new JMenuBar();
private JMenu fileMenu = new JMenu("File");
private JMenuItem newMenuItem = new JMenuItem("New");
private JMenuItem openMenuItem = new JMenuItem("Open");
private JMenuItem saveMenuItem = new JMenuItem("Save");
private JMenuItem exitMenuItem = new JMenuItem("Exit");
public SimpleNotepad() {
setTitle("Simple Notepad");
// Set the size of the window
setSize(640, 480);
// Set the location of the window
setLocationRelativeTo(null);
// Add the text area to the content pane
getContentPane().add(textArea);
// Add the menu bar to the window
setJMenuBar(menuBar);
// Add the file menu to the menu bar
menuBar.add(fileMenu);
// Add the menu items to the file menu
fileMenu.add(newMenuItem);
fileMenu.add(openMenuItem);
fileMenu.add(saveMenuItem);
fileMenu.addSeparator();
fileMenu.add(exitMenuItem);
// Add the action listeners to the menu items
newMenuItem.addActionListener(this);
openMenuItem.addActionListener(this);
saveMenuItem.addActionListener(this);
exitMenuItem.addActionListener(this);
// Show the window
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
// If the user clicks the "New" menu item
if (e.getSource() == newMenuItem) {
// Clear the text area
textArea.setText("");
}
// If the user clicks the "Open" menu item
else if (e.getSource() == openMenuItem) {
// Create a file chooser dialog
JFileChooser fileChooser = new JFileChooser();
// Show the dialog
int result = fileChooser.showOpenDialog(this);
// If the user clicks the "Open" button
if (result == JFileChooser.APPROVE_OPTION) {
// Get the selected file
File file = fileChooser.getSelectedFile();
try {
// Open the file and read its contents into the text area
BufferedReader reader = new BufferedReader(new FileReader(file));
String line = null;
while ((line = reader.readLine()) != null) {
textArea.append(line + "\n");
}
reader.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
// If the user clicks the "Save" menu item
else if (e.getSource() == saveMenuItem) {
// Create a file chooser dialog
JFileChooser fileChooser = new JFileChooser();
// Show the dialog
int result = fileChooser.showSaveDialog(this);
// If the user clicks the "Save" button
if (result == JFileChooser.APPROVE_OPTION) {
// Get the selected file
File file = fileChooser.getSelectedFile();
try {
// Save the contents of the text area to the file
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
writer.write(textArea.getText());
writer.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
// If the user clicks the "Exit" menu item
else if (e.getSource() == exitMenuItem) {
// Exit the application
System.exit(0);
}
}
public static void main(String[] args) {
new SimpleNotepad();
}
}
```
这个记事本GUI包括了菜单栏和一个文本编辑区域,并且可以实现新建、打开、保存和退出功能。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
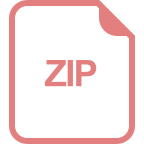
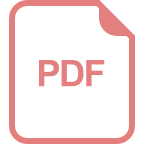
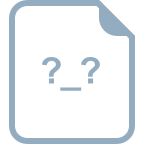















