main.py: error: argument --dataset: expected one argument
时间: 2024-05-04 11:21:17 浏览: 38
这个错误信息看起来是在运行 Python 程序时出现的。它表明程序主入口 `main.py` 指定了一个 `--dataset` 参数,但是这个参数需要接收一个值,而你没有给出这个值。
解决这个问题的方法是,在运行 `main.py` 程序时,指定一个参数值,例如:
```
python main.py --dataset my_dataset
```
其中 `my_dataset` 是你的数据集名称,可以根据实际情况进行修改。如果你不知道应该给出什么值,可以查看程序文档或者询问程序开发者。
相关问题
train.py: error: argument --gpus: expected one argument
这个错误提示是因为在运行 train.py 脚本时,没有为 --gpus 参数指定参数值。请检查你的命令行是否正确输入了参数及其值。
例如,如果你想要在 2 个 GPU 上训练模型,可以使用以下命令:
```
python train.py --gpus 2
```
请注意,具体的参数设置可能因你所使用的训练框架和版本而有所不同。如果你仍然无法解决这个问题,请提供更多的上下文信息和代码,以便我们更好地帮助你。
val.py: error: argument --task: expected one argument
这个错误提示表明你在运行 `val.py` 脚本时,没有给 `--task` 参数传递一个参数值。你需要根据你的具体情况,给 `--task` 参数传递一个参数值。例如:
```
python val.py --task my_task
```
其中 `my_task` 是你要运行的任务名称。请注意,这只是一个示例,你需要根据你的具体情况进行修改。
相关推荐
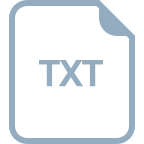
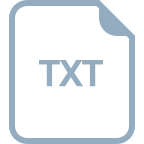
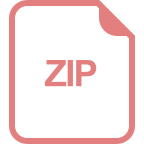












