编写程序建立一个单向链表。链表结点中的数据为从键盘输入的一个宇符串,但要求将该串字符按由小到大的顺序组 在链表中。
时间: 2024-02-11 13:04:20 浏览: 86
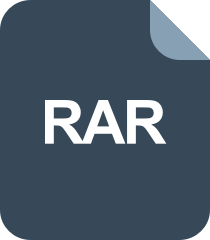
我编写的实现单链表的程序
好的,以下是用C语言实现建立单向链表的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Node {
char data[50];
struct Node *next;
};
void insertNode(struct Node **head_ref, char data[]);
void printList(struct Node *node);
int main() {
struct Node *head = NULL;
char input[50];
printf("请输入一串字符串,以回车结束:\n");
scanf("%s", input);
while (strcmp(input, "exit") != 0) {
insertNode(&head, input);
scanf("%s", input);
}
printf("按顺序输出链表中的字符串:\n");
printList(head);
return 0;
}
void insertNode(struct Node **head_ref, char data[]) {
struct Node *new_node = (struct Node*)malloc(sizeof(struct Node));
strcpy(new_node->data, data);
new_node->next = NULL;
struct Node *current = *head_ref;
if (*head_ref == NULL || strcmp((*head_ref)->data, data) > 0) {
new_node->next = *head_ref;
*head_ref = new_node;
} else {
while (current->next != NULL && strcmp(current->next->data, data) < 0) {
current = current->next;
}
new_node->next = current->next;
current->next = new_node;
}
}
void printList(struct Node *node) {
while (node != NULL) {
printf("%s ", node->data);
node = node->next;
}
printf("\n");
}
```
这段代码实现了一个按照字符串由小到大顺序组成的单向链表。我们定义了一个 `Node` 结构体,其中包含了一个字符串数据 `data` 和指向下一个结点的指针 `next`。在 `main` 函数中,我们通过循环读取输入的字符串,调用 `insertNode` 函数将其插入到链表中。`insertNode` 函数实现了按照字符串由小到大的顺序将结点插入到链表中。最后,我们调用 `printList` 函数按顺序输出链表中的字符串。
阅读全文
相关推荐
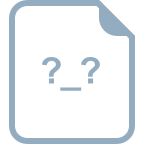












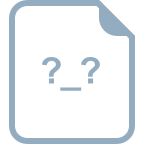
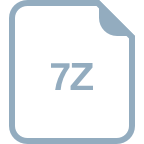
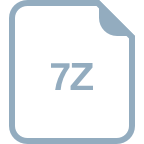