recyclerview 循环滚动代码
时间: 2023-10-17 07:05:38 浏览: 34
使用RecyclerView实现循环滚动,需要对LayoutManager进行定制。以下是示例代码:
```java
public class LoopLayoutManager extends LinearLayoutManager {
private boolean isLoop;
public LoopLayoutManager(Context context, boolean isLoop) {
super(context);
this.isLoop = isLoop;
}
@Override
public int scrollHorizontallyBy(int dx, RecyclerView.Recycler recycler, RecyclerView.State state) {
int scrolled = super.scrollHorizontallyBy(dx, recycler, state);
if (isLoop && scrolled == 0) {
scrolled = scrollLoopHorizontallyBy(dx, recycler, state);
}
return scrolled;
}
private int scrollLoopHorizontallyBy(int dx, RecyclerView.Recycler recycler, RecyclerView.State state) {
int width = getWidth();
if (width <= 0) {
return 0;
}
int totalWidth = getItemCount() * width;
int scrollOffset = getPaddingLeft() - dx;
if (scrollOffset < 0) {
scrollOffset += totalWidth;
} else if (scrollOffset > totalWidth - width) {
scrollOffset -= totalWidth;
}
offsetChildrenHorizontal(-scrollOffset);
return dx;
}
@Override
public int scrollVerticallyBy(int dy, RecyclerView.Recycler recycler, RecyclerView.State state) {
int scrolled = super.scrollVerticallyBy(dy, recycler, state);
if (isLoop && scrolled == 0) {
scrolled = scrollLoopVerticallyBy(dy, recycler, state);
}
return scrolled;
}
private int scrollLoopVerticallyBy(int dy, RecyclerView.Recycler recycler, RecyclerView.State state) {
int height = getHeight();
if (height <= 0) {
return 0;
}
int totalHeight = getItemCount() * height;
int scrollOffset = getPaddingTop() - dy;
if (scrollOffset < 0) {
scrollOffset += totalHeight;
} else if (scrollOffset > totalHeight - height) {
scrollOffset -= totalHeight;
}
offsetChildrenVertical(-scrollOffset);
return dy;
}
@Override
public void onLayoutChildren(RecyclerView.Recycler recycler, RecyclerView.State state) {
super.onLayoutChildren(recycler, state);
if (isLoop && getItemCount() > 0) {
scrollToPosition(0);
}
}
@Override
public void scrollToPosition(int position) {
if (isLoop && getItemCount() > 0) {
position = position % getItemCount();
}
super.scrollToPosition(position);
}
@Override
public void smoothScrollToPosition(RecyclerView recyclerView, RecyclerView.State state, int position) {
if (isLoop && getItemCount() > 0) {
position = position % getItemCount();
}
super.smoothScrollToPosition(recyclerView, state, position);
}
}
```
在使用RecyclerView时,使用上述定制LayoutManager即可实现循环滚动。例如:
```java
RecyclerView recyclerView = findViewById(R.id.recycler_view);
LoopLayoutManager layoutManager = new LoopLayoutManager(this, true);
recyclerView.setLayoutManager(layoutManager);
```
其中,第二个参数`isLoop`表示是否开启循环滚动。
相关推荐
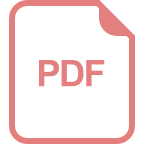
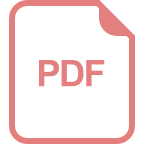
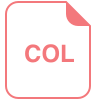













