用cplusplus读取Deploy: input_shape: - -1 - 3 - 352 - 288
时间: 2024-05-08 10:17:52 浏览: 10
这是一个YAML格式的文本,可以使用第三方库如YAML-CPP来读取。
以下是一个示例代码:
```cpp
#include <iostream>
#include <yaml-cpp/yaml.h>
int main() {
std::string yamlText = "Deploy:\n input_shape:\n - -1\n - 3\n - 352\n - 288\n";
YAML::Node root = YAML::Load(yamlText);
std::cout << root["Deploy"]["input_shape"][0].as<int>() << std::endl; // 输出 -1
std::cout << root["Deploy"]["input_shape"][1].as<int>() << std::endl; // 输出 3
std::cout << root["Deploy"]["input_shape"][2].as<int>() << std::endl; // 输出 352
std::cout << root["Deploy"]["input_shape"][3].as<int>() << std::endl; // 输出 288
return 0;
}
```
相关问题
编译sdk动态库但对外接口不用std::__ndk1
如果您想编译 SDK 动态库,并且对外接口不使用 `std::__ndk1`,您可以按照以下步骤进行:
1. 在您的代码中,使用 `extern "C"` 将函数声明包围起来,例如:
```cpp
#ifdef __cplusplus
extern "C" {
#endif
int my_function(int arg1, int arg2);
#ifdef __cplusplus
}
#endif
```
这将在 C++ 代码中指定函数使用 C 语言的调用约定。
2. 在编译时,将 `-fPIC` 和 `-shared` 选项传递给编译器,例如:
```
g++ -fPIC -shared -o libmylibrary.so mycode.cpp
```
使用 `-fPIC` 选项将代码编译为位置无关代码,以便在动态链接时能够正确地解析符号(函数和全局变量)。使用 `-shared` 选项将代码编译为动态链接库。
3. 在链接时,使用 `-Wl,-soname` 选项指定动态库的 soname,例如:
```
g++ -fPIC -shared -Wl,-soname,libmylibrary.so.1 -o libmylibrary.so.1.0.0 mycode.cpp
```
这将生成一个名为 `libmylibrary.so.1.0.0` 的动态库,并将其 soname 指定为 `libmylibrary.so.1`。
4. 在使用动态库时,需要在链接时指定 `-lmylibrary` 选项,例如:
```
g++ myapp.cpp -L/path/to/lib -lmylibrary
```
这将链接到您的动态库,并使其可用于您的应用程序。
需要注意的是,如果您的代码中使用了特定于 NDK 的 API 或库,则无法在不依赖 NDK 的情况下进行编译。
用cplusplus实现提取X-GB-Ver:3.0中的3.0
可以使用正则表达式来提取X-GB-Ver:3.0中的3.0,具体实现如下:
```cpp
#include <iostream>
#include <regex>
#include <string>
int main() {
std::string str = "X-GB-Ver:3.0";
std::regex re("\\d+\\.\\d+");
std::smatch match;
if (std::regex_search(str, match, re)) {
std::cout << match[0] << std::endl;
}
return 0;
}
```
输出结果为:
```
3.0
```
相关推荐
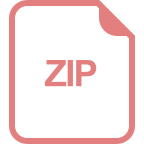
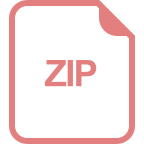












