prim算法生成迷宫
时间: 2023-12-06 11:38:50 浏览: 38
以下是使用prim算法生成迷宫的步骤:
1.创建一个空的网格,其中每个单元格都有四面墙。
2随机选择一个单元格作为当前单元格,并将其标记为已访问。
3.对于当前单元格,随机选择一个未访问的相邻单元格。
4.如果所选单元格尚未被访问,则将当前单元格与所选单元格之间的墙拆除,并将所选单元格标记为已访问。
5.将所选单元格设置为当前单元格。
6.重复步骤3-5,直到所有单元格都被访问。
下面是使用Python实现prim算法生成迷宫的代码:
```python
import random
def generate_maze(width, height):
# 创建一个空的网格
maze = [[{'N': True, 'S': True, 'W': True, 'E': True} for x in range(width)] for y in range(height)]
# 随机选择一个起始单元格
current_x, current_y = random.randint(0, width-1), random.randint(0, height-1)
visited = set([(current_x, current_y)])
# prim算法生成迷宫
while len(visited) < width * height:
neighbors = []
if current_x > 0 and (current_x-1, current_y) not in visited:
neighbors.append(('W', current_x-1, current_y))
if current_x < width-1 and (current_x+1, current_y) not in visited:
neighbors.append(('E', current_x+1, current_y))
if current_y > 0 and (current_x, current_y-1) not in visited:
neighbors.append(('N', current_x, current_y-1))
if current_y < height-1 and (current_x, current_y+1) not in visited:
neighbors.append(('S', current_x, current_y+1))
if not neighbors:
current_x, current_y = visited.pop()
continue
direction, next_x, next_y = random.choice(neighbors)
maze[current_y][current_x][direction] = False
maze[next_y][next_x][{'N': 'S', 'S': 'N', 'W': 'E', 'E': 'W'}[direction]] = False
current_x, current_y = next_x, next_y
visited.add((current_x, current_y))
return maze
```
相关推荐
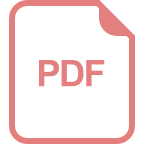
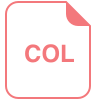
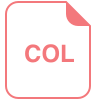
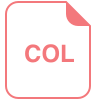











