用C++写一个使用Prim算法生成迷宫的程序,要求墙为单元格且打印迷宫时用在■填充。另外要求自定义起点与终点,要求起点与终点一定要存在通路
时间: 2024-05-09 15:15:58 浏览: 96
以下是使用Prim算法生成迷宫的C++程序,包含自定义起点与终点,并保证起点与终点存在通路。墙为单元格,迷宫以■填充。
```
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
using namespace std;
const int WALL = -1; // 墙
const int PATH = 0; // 路径
const int START = 1; // 起点
const int END = 2; // 终点
class Maze {
public:
Maze(int w, int h, int sx, int sy, int ex, int ey);
void print();
private:
int width; // 迷宫宽度
int height; // 迷宫高度
int start_x; // 起点 x 坐标
int start_y; // 起点 y 坐标
int end_x; // 终点 x 坐标
int end_y; // 终点 y 坐标
vector<vector<int>> maze; // 迷宫
void initMaze(); // 初始化迷宫
void generateMaze(); // 生成迷宫
};
Maze::Maze(int w, int h, int sx, int sy, int ex, int ey) {
width = w;
height = h;
start_x = sx;
start_y = sy;
end_x = ex;
end_y = ey;
initMaze();
generateMaze();
}
void Maze::initMaze() {
maze.resize(height);
for (int i = 0; i < height; i++) {
maze[i].resize(width);
for (int j = 0; j < width; j++) {
if (i == 0 || i == height - 1 || j == 0 || j == width - 1)
maze[i][j] = WALL;
else
maze[i][j] = PATH;
}
}
maze[start_y][start_x] = START;
maze[end_y][end_x] = END;
}
void Maze::generateMaze() {
srand(time(nullptr));
vector<pair<int, int>> frontier;
frontier.push_back(make_pair(start_y, start_x));
while (!frontier.empty()) {
int randIndex = rand() % frontier.size();
int y = frontier[randIndex].first;
int x = frontier[randIndex].second;
frontier.erase(frontier.begin() + randIndex);
if (maze[y][x] == WALL) {
maze[y][x] = PATH;
if (y - 2 > 0 && maze[y - 2][x] == WALL) {
maze[y - 1][x] = PATH;
frontier.push_back(make_pair(y - 2, x));
}
if (x - 2 > 0 && maze[y][x - 2] == WALL) {
maze[y][x - 1] = PATH;
frontier.push_back(make_pair(y, x - 2));
}
if (y + 2 < height - 1 && maze[y + 2][x] == WALL) {
maze[y + 1][x] = PATH;
frontier.push_back(make_pair(y + 2, x));
}
if (x + 2 < width - 1 && maze[y][x + 2] == WALL) {
maze[y][x + 1] = PATH;
frontier.push_back(make_pair(y, x + 2));
}
}
}
}
void Maze::print() {
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (maze[i][j] == WALL)
cout << "■";
else if (maze[i][j] == PATH)
cout << " ";
else if (maze[i][j] == START)
cout << "S";
else if (maze[i][j] == END)
cout << "E";
}
cout << endl;
}
}
int main() {
Maze maze(21, 21, 1, 1, 19, 19);
maze.print();
return 0;
}
```
使用示例:
```
Maze maze(21, 21, 1, 1, 19, 19);
maze.print();
```
其中,构造函数的参数依次为迷宫宽度、迷宫高度、起点x坐标、起点y坐标、终点x坐标、终点y坐标。在示例中,生成21x21的迷宫,并定义起点为(1,1),终点为(19,19)。
阅读全文
相关推荐
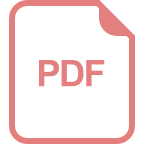
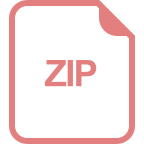






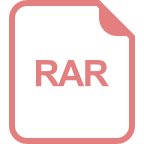
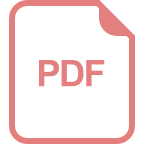
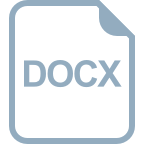
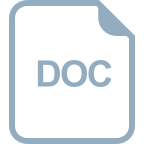
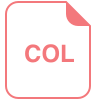

