c++怎么获取字符串中重复的字符和重复字符的出现次数
时间: 2024-11-18 18:16:20 浏览: 13
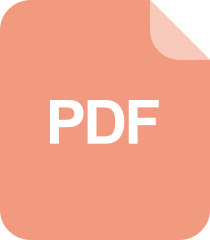
C++实现从输入中读取字符串
在 C++ 中,获取字符串中重复的字符以及它们的出现次数,可以采用哈希表(如 `std::unordered_map`)来存储字符及其对应的计数。下面是一个简单的步骤:
1. 定义一个函数,比如 `findDuplicateChars(string str)`,接受一个字符串作为输入。
2. 创建一个空的 `unordered_map<char, int>`,用于存储字符和出现次数。
3. 遍历字符串中的每个字符 `c`:
a. 如果 `c` 已经在哈希表中,则将其计数值加一;否则,添加到哈希表并设置计数为 1。
4. 再次遍历哈希表,找出计数大于 1 的字符,即重复的字符。
这里是伪代码形式:
```cpp
#include <string>
#include <unordered_map>
std::pair<std::string, std::map<char, int>> findDuplicateChars(const std::string& str) {
std::unordered_map<char, int> charCount;
for (char c : str) {
if (charCount.find(c) != charCount.end()) {
charCount[c]++;
} else {
charCount[c] = 1;
}
}
std::vector<std::pair<char, int>> duplicates;
for (const auto &entry : charCount) {
if (entry.second > 1) {
duplicates.push_back(entry);
}
}
return {duplicates, charCount}; // 返回重复字符的集合和整体计数
}
// 使用示例
int main() {
std::string input = "hello world";
auto result = findDuplicateChars(input);
for (auto &duplicate : result.first) {
cout << "Character '" << duplicate.first << "' appears " << duplicate.second << " times.\n";
}
return 0;
}
```
阅读全文
相关推荐
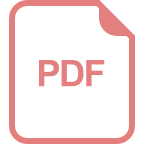
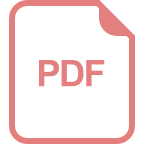
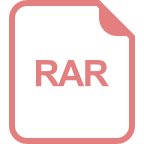
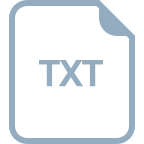
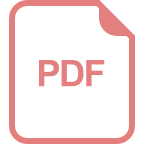
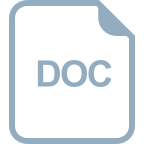
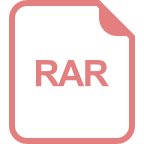
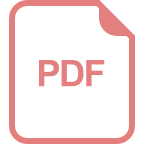
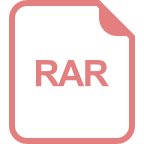
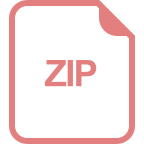
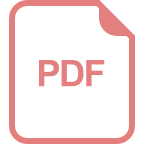
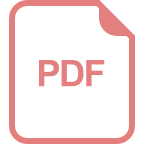
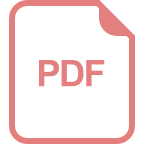
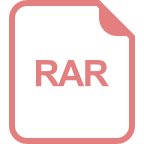
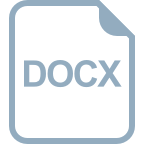
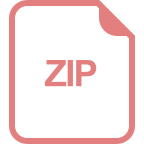
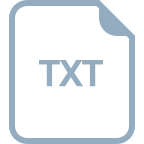