判断字符串是否是回文C++
时间: 2023-12-01 22:42:05 浏览: 86
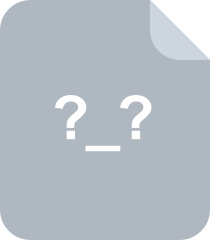
判断字符串是否是回文

以下是C++判断字符串是否是回文的代码示例:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[101];
cin >> str;
int len = strlen(str);
bool isPalindrome = true;
for (int i = 0; i < len / 2; i++) {
if (str[i] != str[len - i - 1]) {
isPalindrome = false;
break;
}
}
if (isPalindrome) {
cout << "yes" << endl;
} else {
cout << "no" << endl;
}
return 0;
}
```
该代码首先读入一个字符串,然后使用一个布尔变量`isPalindrome`来记录该字符串是否是回文。接着使用一个循环遍历字符串的前一半字符,判断其是否与后一半字符对应位置的字符相同,如果不同则将`isPalindrome`设为`false`并跳出循环。最后根据`isPalindrome`的值输出结果。
阅读全文
相关推荐
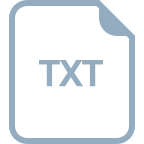















