c++用指针数组输入并判断字符串是否是回文序列
时间: 2023-12-17 15:05:26 浏览: 109
可以按照以下步骤来实现:
1. 定义一个指针数组,存储字符串。
2. 从控制台输入字符串,使用 `gets()` 或 `fgets()` 函数,将输入的字符串存储到指针数组中。
3. 定义两个指针,分别指向字符串的头和尾。
4. 使用 `while` 循环,比较两个指针所指向的字符是否相等,如果相等,则将两个指针向中间移动,继续比较下一个字符,直到两个指针相遇或者交错。
5. 如果两个指针相遇或者交错,则说明该字符串是回文序列。
完整的代码如下:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main()
{
const int MAX_SIZE = 100;
char* str[MAX_SIZE]; // 定义指针数组
char buf[MAX_SIZE];
cout << "请输入一个字符串: ";
gets(buf); // 从控制台输入字符串
// 将字符串存储到指针数组中
int len = strlen(buf);
for (int i = 0; i < len; i++)
{
str[i] = &buf[i];
}
// 判断字符串是否是回文序列
char* left = str[0];
char* right = str[len - 1];
while (left < right)
{
if (*left != *right)
{
cout << "不是回文序列" << endl;
return 0;
}
left++;
right--;
}
cout << "是回文序列" << endl;
return 0;
}
```
阅读全文
相关推荐
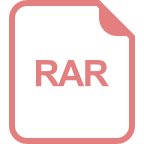
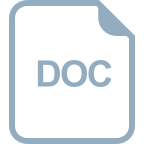










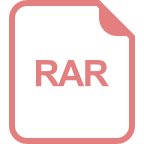
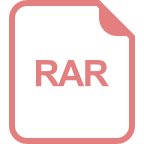
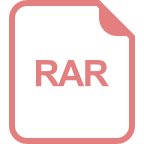
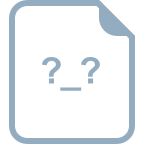