esp32向手机发送数据
时间: 2023-10-23 22:02:44 浏览: 150
ESP32是一款非常流行的物联网开发板,它具有内置的Wi-Fi和蓝牙模块,可以方便地与手机进行通信。如果想要通过ESP32向手机发送数据,可以采用以下步骤:
1. ESP32连接到Wi-Fi网络:首先,将ESP32连接到一个可用的Wi-Fi网络,这样它才能与手机进行通信。通过编写适当的代码,可以将ESP32配置为连接到指定的Wi-Fi网络,并获取一个IP地址。
2. 开启蓝牙通信:ESP32还具有蓝牙功能,可以与手机进行蓝牙通信。首先,需要在ESP32上启用蓝牙模块,并使其可见。然后,使用蓝牙库函数,例如ESP-IDF中的BluetoothSerial库函数,可以实现与手机的蓝牙连接。
3. 发送数据到手机:一旦ESP32连接到Wi-Fi网络和手机的蓝牙上,就可以通过编写代码将数据从ESP32发送到手机。可以将要发送的数据封装在一个适当的数据包中,例如JSON格式。然后,使用适当的通信协议,例如HTTP或WebSocket,将数据发送到手机的指定地址与端口上。
4. 手机接收数据:在手机上,可以编写一个应用程序来接收从ESP32发送的数据。可以使用适当的网络通信库、蓝牙库或者其他相关的库函数来接收数据。一旦接收到数据,可以对其进行解析和处理,例如显示在应用程序的界面上或执行特定的操作。
总结起来,实现ESP32向手机发送数据的步骤包括连接到Wi-Fi网络、开启蓝牙通信、发送数据到手机以及手机接收数据。通过编写适当的代码,可以实现ESP32与手机之间的通信,实现数据的传输和交换。这为物联网应用提供了更多的可能性和灵活性。
相关问题
esp32向手机发送数据的代码
以下是使用 ESP32 和 Bluetooth LE 向手机发送数据的示例代码:
```c
#include <BLEDevice.h>
#include <BLEUtils.h>
#include <BLEServer.h>
BLECharacteristic *pCharacteristic;
bool deviceConnected = false;
bool oldDeviceConnected = false;
uint8_t txValue = 0;
class MyServerCallbacks: public BLEServerCallbacks {
void onConnect(BLEServer* pServer) {
deviceConnected = true;
};
void onDisconnect(BLEServer* pServer) {
deviceConnected = false;
}
};
void setup() {
Serial.begin(115200);
BLEDevice::init("ESP32 BLE Example");
BLEServer *pServer = BLEDevice::createServer();
pServer->setCallbacks(new MyServerCallbacks());
BLEService *pService = pServer->createService(BLEUUID((uint16_t)0x180F));
pCharacteristic = pService->createCharacteristic(
BLEUUID((uint16_t)0x2A19),
BLECharacteristic::PROPERTY_READ |
BLECharacteristic::PROPERTY_NOTIFY
);
pCharacteristic->addDescriptor(new BLE2902());
pService->start();
BLEAdvertising *pAdvertising = BLEDevice::getAdvertising();
pAdvertising->addServiceUUID(pService->getUUID());
pAdvertising->setScanResponse(true);
pAdvertising->setMinPreferred(0x06);
pAdvertising->setMinPreferred(0x12);
BLEDevice::startAdvertising();
}
void loop() {
if (deviceConnected) {
txValue++;
pCharacteristic->setValue(&txValue, 1);
pCharacteristic->notify();
delay(10);
}
if (deviceConnected != oldDeviceConnected) {
oldDeviceConnected = deviceConnected;
if (deviceConnected) {
Serial.println("Device connected");
} else {
Serial.println("Device disconnected");
}
}
}
```
在这个例子中,我们使用了 ESP32 的 Bluetooth LE 模块。首先,我们初始化 BLE 设备并创建一个 BLE 服务器和服务。然后,我们在服务中创建了一个特征,该特征可以读取和通知。在主循环中,我们检查设备是否连接。如果是,我们增加一个计数器并将其发送到手机。延迟 10 毫秒以避免发送太多数据。最后,我们打印设备是否连接的状态。
esp32蓝牙向手机发送数据Python
使用ESP32的蓝牙模块与手机进行通信,可以使用Python的Bluetooth模块来实现。下面是一个简单的例子,演示了如何使用ESP32的蓝牙模块向手机发送数据:
```python
import bluetooth
# 设置ESP32的蓝牙地址
esp32_mac = 'xx:xx:xx:xx:xx:xx'
# 连接到ESP32的蓝牙设备
sock = bluetooth.BluetoothSocket(bluetooth.RFCOMM)
sock.connect((esp32_mac, 1))
# 发送数据到ESP32
data = 'Hello, ESP32!'
sock.send(data)
# 关闭蓝牙连接
sock.close()
```
在上面的代码中,我们首先设置了ESP32的蓝牙地址。然后,我们使用Python的`bluetooth`模块建立一个蓝牙连接,并将数据发送到ESP32。最后,我们关闭蓝牙连接。
请注意,`bluetooth`模块的使用可能会因操作系统和Python版本的差异而有所不同。如果您遇到问题,请参考官方文档或其他资源。
相关推荐
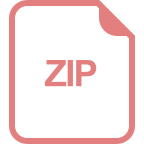












