对称算法和非对称算法
时间: 2023-11-25 11:50:23 浏览: 43
对称算法和非对称算法是两种常见的加密算法。
对称算法是指加密和解密使用相同的密钥,常见的对称算法有DES、3DES、AES、Blowfish、Twofish等。对称算法的优点是加密解密速度快,缺点是密钥管理困难,密钥容易被泄露,安全性相对较低。
非对称算法是指加密和解密使用不同的密钥,常见的非对称算法有RSA、DSA、ECC等。非对称算法的优点是密钥管理方便,安全性相对较高,缺点是加密解密速度慢。
下面是对称算法和非对称算法的代码实例:
对称算法AES加密解密:
```python
from Crypto.Cipher import AES
import base64
# 加密函数
def aes_encrypt(key, data):
cipher = AES.new(key.encode('utf-8'), AES.MODE_ECB)
encrypted_data = cipher.encrypt(data.encode('utf-8'))
return base64.b64encode(encrypted_data).decode('utf-8')
# 解密函数
def aes_decrypt(key, encrypted_data):
cipher = AES.new(key.encode('utf-8'), AES.MODE_ECB)
decrypted_data = cipher.decrypt(base64.b64decode(encrypted_data.encode('utf-8')))
return decrypted_data.decode('utf-8')
# 测试
key = '1234567890123456'
data = 'hello world'
encrypted_data = aes_encrypt(key, data)
print('加密后的数据:', encrypted_data)
decrypted_data = aes_decrypt(key, encrypted_data)
print('解密后的数据:', decrypted_data)
```
非对称算法RSA加密解密:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_v1_5
import base64
# 生成密钥对
key = RSA.generate(1024)
# 获取公钥和私钥
public_key = key.publickey().exportKey()
private_key = key.exportKey()
# 加密函数
def rsa_encrypt(public_key, data):
cipher = PKCS1_v1_5.new(RSA.importKey(public_key))
encrypted_data = cipher.encrypt(data.encode('utf-8'))
return base64.b64encode(encrypted_data).decode('utf-8')
# 解密函数
def rsa_decrypt(private_key, encrypted_data):
cipher = PKCS1_v1_5.new(RSA.importKey(private_key))
decrypted_data = cipher.decrypt(base64.b64decode(encrypted_data.encode('utf-8')), None)
return decrypted_data.decode('utf-8')
# 测试
data = 'hello world'
encrypted_data = rsa_encrypt(public_key, data)
print('加密后的数据:', encrypted_data)
decrypted_data = rsa_decrypt(private_key, encrypted_data)
print('解密后的数据:', decrypted_data)
```
相关推荐
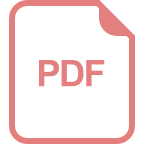














