如何在C++中实现向网页发送数据的功能?
时间: 2024-11-22 17:37:45 浏览: 22
在C++中,通常不会直接操作HTTP请求发送数据到网页,因为这涉及到网络编程和HTTP库的使用,而C++标准库本身并不包含这样的功能。但你可以通过以下几个步骤来实现这个功能:
1. **第三方库**:使用像`cpprestsdk`(微软提供的)、`cURL`、`Poco`库或是`asio`(异步I/O库)等,它们都提供处理HTTP请求的能力。
```cpp
#include <cpprest/http_client.h>
using namespace web;
using namespace web::http;
// 使用cpprestsdk的例子
HttpClient client;
uri_builder builder("https://example.com/api");
auto request = client.request(methods::POST, builder.to_string());
request.headers().add("Content-Type", "application/json");
json::value body;
body["key"] = "value";
request.set_body(body);
http_response response = await request.send();
```
2. **文件描述符和套接字**:如果你更倾向于底层操作,可以使用`boost.asio`库创建套接字,并手动构造HTTP请求和响应。
3. **CURL API**:
```cpp
#include <curl/curl.h>
CURL *curl;
CURLcode res;
std::string url = "https://example.com/api";
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, url.c_str());
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "data=value");
res = curl_easy_perform(curl);
// 处理结果并关闭连接
curl_easy_cleanup(curl);
}
```
4. **使用现代HTTP客户端库**:如果是在服务器端环境,可以考虑使用像`nlohmann/json`和`RapidJSON`之类的库配合HTTP服务器框架(如`Poco`的Net模块或`CpprestSDK`)。
记得在使用前查看库的文档,了解如何设置正确的请求头、编码数据以及处理返回结果。
阅读全文
相关推荐
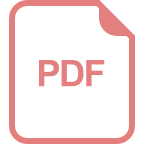
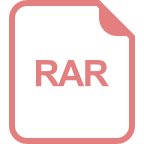
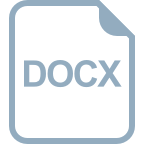
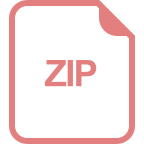
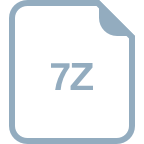
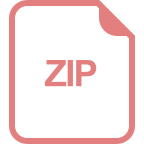
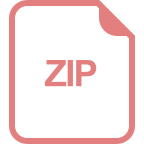
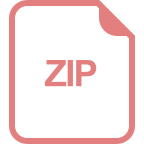
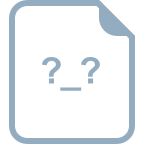
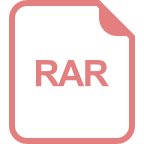
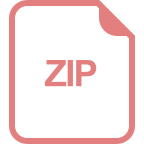
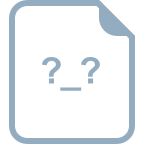
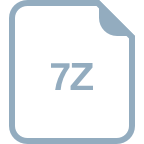
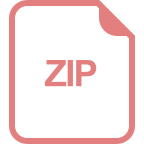
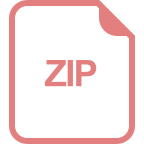
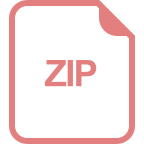
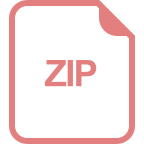