定义一个复数类.包括实部跟虚部成员变量、构造方法、以及两个复数的加法、乘法和比较大小运算符定义
时间: 2024-12-11 13:30:03 浏览: 15
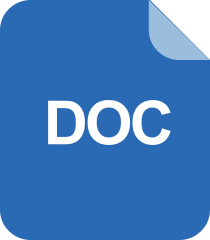
实验五、运算符重载 复数+-*/ 大数+-*/
为了定义一个复数类,我们可以按照面向对象编程的原则,在Python中创建一个名为`ComplexNumber`的类。这个类将包含实部(`real`)和虚部(`imaginary`)两个私有成员变量,以及对应的构造方法,用于初始化复数。此外,我们将提供加法、乘法和比较大小的方法。
```python
class ComplexNumber:
def __init__(self, real=0, imaginary=0):
"""
构造方法,接受实部和虚部作为参数,如果省略则默认为0
"""
self.__real = real
self.__imaginary = imaginary
# 访问器方法,用于获取或设置复数的实部和虚部
@property
def real(self):
return self.__real
@real.setter
def real(self, value):
self.__real = value
@property
def imaginary(self):
return self.__imaginary
@imaginary.setter
def imaginary(self, value):
self.__imaginary = value
# 加法方法
def add(self, other):
new_real = self.real + other.real
new_imaginary = self.imaginary + other.imaginary
return ComplexNumber(new_real, new_imaginary)
# 乘法方法
def multiply(self, other):
real_part = self.real * other.real - self.imaginary * other.imaginary
imag_part = self.real * other.imaginary + self.imaginary * other.real
return ComplexNumber(real_part, imag_part)
# 比较大小的运算符重载
def __lt__(self, other):
return (self.real, self.imaginary) < (other.real, other.imaginary)
def __le__(self, other):
return (self.real, self.imaginary) <= (other.real, other.imaginary)
def __gt__(self, other):
return (self.real, self.imaginary) > (other.real, other.imaginary)
def __ge__(self, other):
return (self.real, self.imaginary) >= (other.real, other.imaginary)
def __eq__(self, other):
return (self.real, self.imaginary) == (other.real, other.imaginary)
def __ne__(self, other):
return (self.real, self.imaginary) != (other.real, other.imaginary)
# 示例
c1 = ComplexNumber(3, 4)
c2 = ComplexNumber(1, 2)
print("c1 =", c1)
print("c2 =", c2)
print("c1 + c2 =", c1.add(c2))
print("c1 * c2 =", c1.multiply(c2))
```
阅读全文
相关推荐
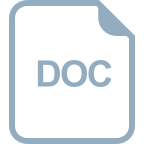
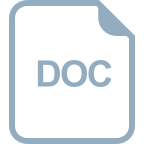

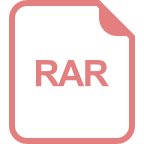
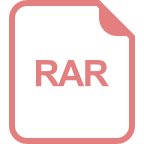
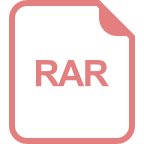
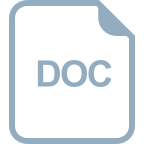
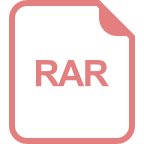
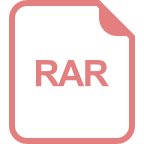
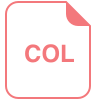


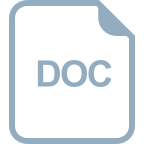
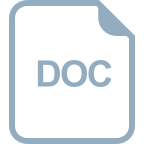
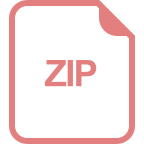