如何在C++中设计一个支持基本运算(如加法、减法、乘法和除法)的复数类?
时间: 2024-10-24 12:14:33 浏览: 46
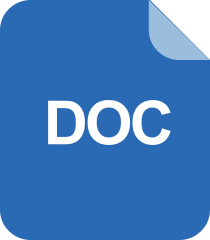
两个高次多项式加法和乘法运算课程设计
在C++中设计一个支持基本运算的复数类,可以按照以下步骤进行:
1. **定义复数结构体**:
首先,创建一个名为`Complex`的结构体,包含两个成员变量,分别表示实部(real)和虚部(imaginary)。
```cpp
struct Complex {
double real;
double imaginary;
};
```
2. **构造函数**: 提供默认构造函数、接受实部和虚部的构造函数以及复制构造函数。
```cpp
// 默认构造函数
Complex() : real(0), imaginary(0) {}
// 接受实部和虚部
Complex(double r, double i = 0) : real(r), imaginary(i) {}
// 复制构造函数
Complex(const Complex& c) : real(c.real), imaginary(c.imaginary) {}
```
3. **运算符重载**: 对于加法、减法、乘法和除法,我们需要提供运算符重载的方法。这里以加法为例:
```cpp
// 加法
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imaginary + other.imaginary);
}
// 减法
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imaginary - other.imaginary);
}
// 乘法
Complex operator*(const Complex& other) const {
double newReal = real * other.real - imaginary * other.imaginary;
double newImaginary = real * other.imaginary + imaginary * other.real;
return Complex(newReal, newImaginary);
}
// 除法(假设其他不为零)
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imaginary * other.imaginary;
double newReal = (real * other.real + imaginary * other.imaginary) / denominator;
double newImaginary = (imaginary * other.real - real * other.imaginary) / denominator;
return Complex(newReal, newImaginary);
}
```
4. **友元声明**: 如果想让其他类能够访问复数内部的数据,需要将当前类声明为其他类的友元。
```cpp
class Complex; // 将Complex声明为友元
class SomeClass {
public:
friend std::ostream& operator<<(std::ostream& os, const Complex& c); // 友元流操作
};
```
5. **显示复数**:
为了方便打印,可以提供一个流操作符重载,使得`std::cout << complexObj << std::endl;`能够正常工作。
```cpp
std::ostream& operator<<(std::ostream& os, const Complex& c) {
return os << "(" << c.real << " + " << c.imaginary << "i)";
}
```
现在你有了一个简单的复数类,可以进行基本的数学运算了。使用这个类的时候,只需要创建对象并使用上面定义的操作符即可。
阅读全文
相关推荐
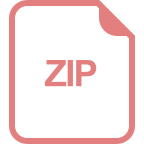
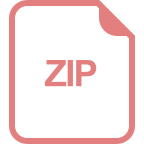
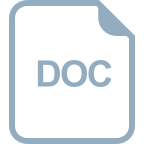













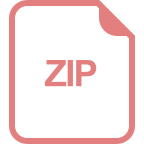
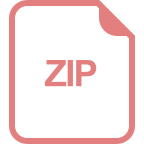