需要一个能根据作物的单价,亩产量,种植成本和年销量计算出最经济的种植方法的c++代码
时间: 2024-09-08 11:01:31 浏览: 20
为了计算最经济的种植方法,我们可以编写一个简单的C++程序,该程序将包含一个函数,用于根据给定的单价、亩产量、种植成本和年销量来计算总利润,并找出最优解。以下是一个可能的实现方式:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
struct CropInfo {
double unitPrice; // 单价
double yieldPerMu; // 每亩产量
double costPerMu; // 每亩种植成本
int annualSales; // 年销量
};
double calculateProfit(const CropInfo& crop, int mu) {
double totalRevenue = crop.unitPrice * crop.yieldPerMu * mu;
double totalCost = crop.costPerMu * mu;
return totalRevenue - totalCost; // 利润 = 总收入 - 总成本
}
int findMostEconomicalMu(const CropInfo& crop, int minMu, int maxMu) {
double maxProfit = 0.0;
int mostEconomicalMu = minMu;
for (int mu = minMu; mu <= maxMu; ++mu) {
double profit = calculateProfit(crop, mu);
if (profit > maxProfit) {
maxProfit = profit;
mostEconomicalMu = mu;
}
}
return mostEconomicalMu;
}
int main() {
// 假设我们有以下作物信息
CropInfo crop = {10.0, 500.0, 200.0, 10000}; // 单价10元,亩产量500公斤,种植成本200元/亩,年销量10000公斤
// 假设我们的种植规模范围是1亩到100亩
int minMu = 1;
int maxMu = 100;
// 找出最经济的种植亩数
int mostEconomicalMu = findMostEconomicalMu(crop, minMu, maxMu);
std::cout << "最经济的种植方法是种植" << mostEconomicalMu << "亩。" << std::endl;
return 0;
}
```
这段代码定义了一个`CropInfo`结构体来存储作物的相关信息。`calculateProfit`函数用于计算给定亩数下的总利润。`findMostEconomicalMu`函数则尝试不同亩数的组合,找出能够产生最大利润的亩数。
相关推荐
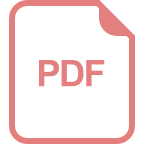
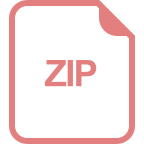
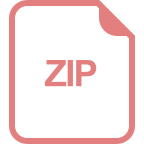














