用面向对象的思维设计相关类,从而实现直线与直线、直线与圆、直线与矩形的交点。 要求各给出每个案例的至少一个示例的程序。 备注:直线方程采用 ay+bx+c=0 形式,矩形的四条边平行于坐标轴 x,y。使用C++语言
时间: 2024-10-10 16:02:50 浏览: 25
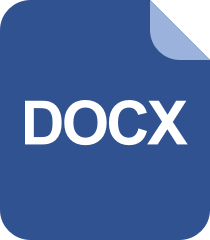
opencv_检测直线、线段、圆、矩形
在面向对象编程中,我们可以创建一些基础几何形状类如`Line`, `Circle`, 和 `Rectangle`,然后为它们各自提供计算与其他形状交点的方法。这里我会简述一下如何设计,并给出每个情况的一个基本示例。由于实际代码可能会比较长,我将简化版的伪代码展示给你。
首先,创建基础类:
```cpp
class Shape {
public:
virtual ~Shape() {}
// 抽象方法,子类需要实现具体的交点计算
virtual std::vector<Point> intersection(Shape* other) const = 0;
};
class Line : public Shape {
private:
double m, b, c; // 直线方程 a*y + b*x + c = 0 的系数
public:
Line(double m, double b, double c) : m(m), b(b), c(c) {}
// ... 实现具体的交点计算(对于两直线)
std::vector<Point> intersection(Line& other) const override;
};
class Circle : public Shape {
private:
Point center; // 圆心
double radius; // 半径
public:
Circle(Point center, double radius) : center(center), radius(radius) {}
// ... 实现具体的交点计算(对于直线与圆)
std::vector<Point> intersection(Line& line) const override;
};
class Rectangle : public Shape {
private:
Point topLeft, bottomRight; // 矩形的顶点坐标
public:
Rectangle(Point topLeft, Point bottomRight) : topLeft(topLeft), bottomRight(bottomRight) {}
// ... 实现具体的交点计算(对于直线与矩形)
std::vector<Point> intersection(Line& line) const override;
};
```
每个类的具体交点计算方法可以基于数学公式,例如:
```cpp
// Line 类的交点计算简化版本
std::vector<Point> Line::intersection(Line& other) const {
// ... 使用高斯消元等方法找到交点,如果不存在,则返回空向量
}
// Circle 类的交点计算简化版本
std::vector<Point> Circle::intersection(Line& line) const {
// ... 计算相切或相交的情况
}
// Rectangle 类的交点计算简化版本
std::vector<Point> Rectangle::intersection(Line& line) const {
// ... 检查线是否垂直于任一边,或者是否穿过矩形内部,等等
}
```
阅读全文
相关推荐
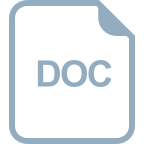
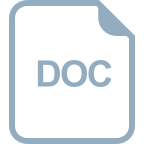
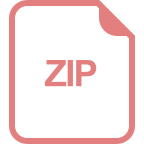


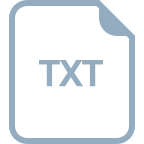
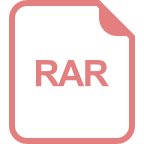
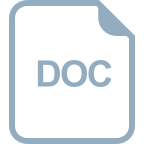
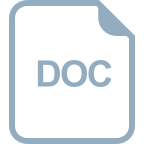
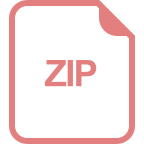
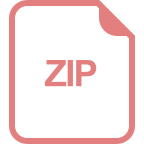
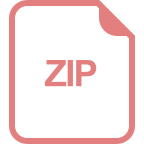
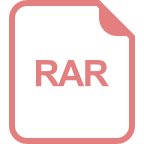
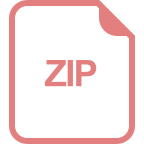