请根据编译原理中的正规式概念,使用C语言编写一个函数,该函数能够匹配一个01串,要求该字符串中0的个数为偶数,而1的个数为奇数。
时间: 2024-11-10 21:20:02 浏览: 28
为了解决这个问题,我们需要先了解正规式(Regular Expression)的基本知识以及C语言中处理字符串的方法。在C语言标准库中,并没有直接支持正则表达式的函数,但我们可以利用递归思想来检查字符串中的0和1的数量。
参考资源链接:[编译原理课后习题与解答解析](https://wenku.csdn.net/doc/55ns7qgffo?spm=1055.2569.3001.10343)
首先,我们可以创建一个递归函数来分别计算字符串中0和1的数量。然后,根据数量是否满足偶数个0和奇数个1的条件来判断字符串是否匹配。
以下是一个可能的C语言实现:
```c
#include <stdio.h>
#include <string.h>
// 递归函数用于计算0的数量
int countZeros(const char *str, int index) {
if (str[index] == '\0') {
return 0;
} else if (str[index] == '0') {
return 1 + countZeros(str, index + 1);
} else {
return countZeros(str, index + 1);
}
}
// 递归函数用于计算1的数量
int countOnes(const char *str, int index) {
if (str[index] == '\0') {
return 0;
} else if (str[index] == '1') {
return 1 + countOnes(str, index + 1);
} else {
return countOnes(str, index + 1);
}
}
// 主函数用于检查字符串是否符合要求
int matchString(const char *str) {
int zeroCount = countZeros(str, 0);
int oneCount = countOnes(str, 0);
// 判断0的数量是否为偶数且1的数量为奇数
return (zeroCount % 2 == 0) && (oneCount % 2 == 1);
}
int main() {
const char *testStr =
参考资源链接:[编译原理课后习题与解答解析](https://wenku.csdn.net/doc/55ns7qgffo?spm=1055.2569.3001.10343)
阅读全文
相关推荐
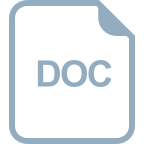
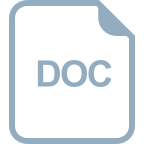
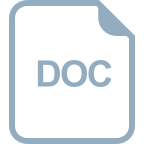
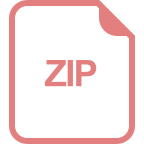
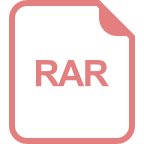
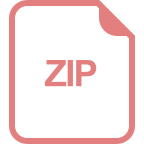
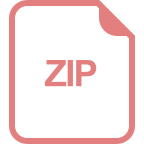
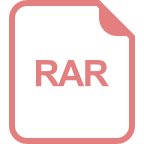
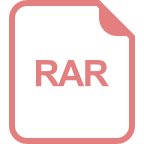
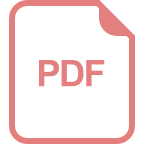
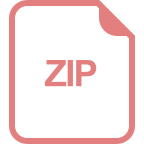
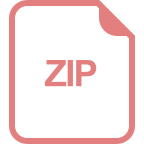
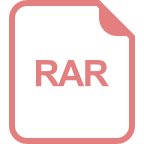
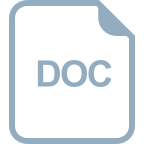
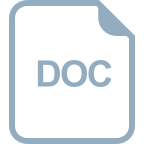
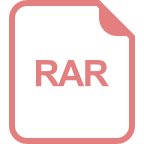
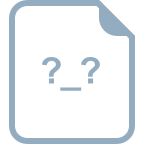
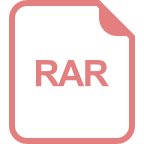
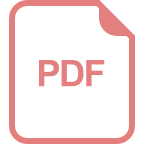