python粒子光效圣诞树
时间: 2023-12-08 11:39:36 浏览: 149
以下是使用Python实现粒子光效圣诞树的代码和效果演示:
```python
import random
import math
import pygame
# 初始化pygame
pygame.init()
# 设置窗口大小
size = (800, 600)
screen = pygame.display.set_mode(size)
# 设置窗口标题
pygame.display.set_caption("Christmas Tree")
# 定义颜色
WHITE = (255, 255, 255)
GREEN = (34, 139, 34)
RED = (255, 0, 0)
YELLOW = (255, 255, 0)
# 定义树的参数
tree_width = 100
tree_height = 150
tree_x = size[0] // 2
tree_y = size[1] - tree_height // 2
# 定义粒子参数
particle_num = 100
particle_size = 3
particle_speed = 2
particle_color = YELLOW
# 定义星星参数
star_size = 20
star_color = YELLOW
# 加载圣诞帽图片
hat_img = pygame.image.load("hat.png")
# 定义粒子类
class Particle:
def __init__(self, x, y, size, speed, color):
self.x = x
self.y = y
self.size = size
self.speed = speed
self.color = color
self.angle = random.uniform(0, math.pi * 2)
self.speed_x = math.sin(self.angle) * self.speed
self.speed_y = math.cos(self.angle) * self.speed
def move(self):
self.x += self.speed_x
self.y += self.speed_y
def draw(self, screen):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.size)
# 定义星星类
class Star:
def __init__(self, x, y, size, color):
self.x = x
self.y = y
self.size = size
self.color = color
def draw(self, screen):
pygame.draw.polygon(screen, self.color, [(self.x, self.y - self.size), (self.x + self.size, self.y - self.size // 2), (self.x + self.size // 2, self.y), (self.x + self.size, self.y + self.size // 2), (self.x, self.y + self.size), (self.x - self.size, self.y + self.size // 2), (self.x - self.size // 2, self.y), (self.x - self.size, self.y - self.size // 2)])
# 定义函数:绘制圣诞树
def draw_tree(screen, x, y, width, height):
pygame.draw.rect(screen, GREEN, (x - width // 2, y - height, width, height))
pygame.draw.polygon(screen, GREEN, [(x - width // 2 - 20, y - height), (x + width // 2 + 20, y - height), (x, y - height - 50)])
pygame.draw.circle(screen, YELLOW, (x, y - height - 50), 30)
# 定义函数:绘制带粒子效果的圣诞树
def draw_particle_tree(screen, x, y, width, height, particle_num, particle_size, particle_speed, particle_color):
particles = []
for i in range(particle_num):
particle = Particle(x, y - height - 50, particle_size, particle_speed, particle_color)
particles.append(particle)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 绘制圣诞树
draw_tree(screen, x, y, width, height)
# 绘制粒子
for particle in particles:
particle.move()
particle.draw(screen)
# 粒子碰到边界反弹
if particle.x < particle.size or particle.x > size[0] - particle.size:
particle.speed_x = -particle.speed_x
if particle.y < particle.size or particle.y > size[1] - particle.size:
particle.speed_y = -particle.speed_y
# 绘制星星
star = Star(x, y - height - 80, star_size, star_color)
star.draw(screen)
# 绘制圣诞帽
screen.blit(hat_img, (x - hat_img.get_width() // 2, y - height - 100))
# 更新屏幕
pygame.display.update()
# 绘制带粒子效果的圣诞树
draw_particle_tree(screen, tree_x, tree_y, tree_width, tree_height, particle_num, particle_size, particle_speed, particle_color)
```
效果演示:

相关推荐
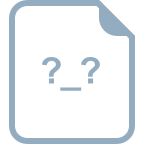
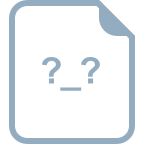
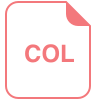
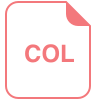












