java poi流根据word模板插入文件
时间: 2023-09-14 09:01:24 浏览: 44
使用Java POI流根据Word模板插入文件可以通过以下步骤实现:
1. 首先,确保已经导入了POI的相关依赖库,可以在Maven中添加以下依赖项来引入POI库:
```xml
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.0</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.0</version>
</dependency>
```
2. 创建一个Word模板文件,其中包含需要填充的占位符,如${name}等。
3. 使用Java代码加载Word模板文件,可以使用`FileInputStream`类来实现:
```java
FileInputStream fis = new FileInputStream("template.docx");
```
4. 创建一个`XWPFDocument`对象,并使用模板文件流初始化它:
```java
XWPFDocument document = new XWPFDocument(fis);
```
5. 获取文档中的段落,可以使用`document.getParagraphs()`方法:
```java
List<XWPFParagraph> paragraphs = document.getParagraphs();
```
6. 遍历段落列表,查找需要替换的占位符,并使用`replaceText`方法将其替换为需要插入的内容:
```java
for (XWPFParagraph paragraph : paragraphs) {
for (XWPFRun run : paragraph.getRuns()) {
String text = run.getText(0);
if (text != null && text.contains("${name}")) {
text = text.replace("${name}", "John Doe"); // 替换为需要插入的内容
run.setText(text, 0);
}
}
}
```
7. 保存更改后的文档,可以使用`FileOutputStream`类来实现:
```java
FileOutputStream fos = new FileOutputStream("output.docx");
document.write(fos);
fos.close();
```
通过上述步骤,可以使用Java POI流根据Word模板插入文件。在遍历段落时,可以根据实际需求进行逻辑修改和扩展,以适应不同的模板和替换要求。
相关推荐
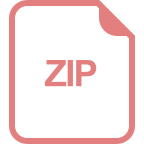
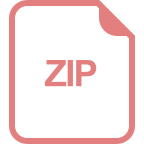














