# 选择排序:对列表中的 字符串长度从小到大排序 list2 = ['aaa', 'bbbbbb', 'cccc', 'ee', 'dddddddd', 'a', '123456789']
时间: 2024-08-13 17:00:52 浏览: 46
选择排序是一种简单的排序算法,它的工作原理是每次从未排序的部分找到最小(或最大,取决于升序还是降序)的元素,并将其放置在已排序部分的末尾。对于给定的字符串列表 `list2`,我们想要按照字符串长度进行升序排列。
以下是选择排序的一个示例步骤:
1. 找到列表中最短的字符串(即最小长度),这里是 'a'。
2. 将该字符串移到已排序部分的起始位置。
3. 从剩余未排序的字符串中找到第二短的字符串,如 '123456789',并放到上一步得到的位置之后。
4. 重复此过程,直到整个列表都排序完毕。
按这个策略操作后,`list2` 就会变成 `['a', 'eee', 'aa', '123456789', 'ccc', 'bbbbbb', 'dddddddd']`。
相关问题
设计字符串类CSTRING,包含私有成员数据p(char *p)。 程序功能:首先读入n个字符串;然后对n个字符串按照升序排列;最后输出排序后的结果。 main函数已给定,提交时只需要提交main函数外的代码部分。 #include<iostream> #include<cstring> using namespace std; //你提交的代码在这里 int main() { int n,i,j; cin>>n; CSTRING t,*cs=new CSTRING[n]; for(i=0;i<n;i++) cin>>cs[i]; for(i=0;i<n-1;i++) for(j=0;j<n-i-1;j++) if(cs[j]>cs[j+1]) { t=cs[j]; cs[j]=cs[j+1]; cs[j+1]=t; } for(i=0;i<n;i++) cout<<"("<<i+1<<") "<<cs[i]<<endl; delete[] cs; return 0; } Input 第1行为1个正整数n,表示字符串的个数n; 第2行包含n个字符串,相互之间用空格隔开。 Output 输出n个字符串排序后的结果,具体格式详见样例。输入5 ccccc bbbbbb rrrrr aaaaaaaa ddddd输出(1) aaaaaaaa (2) bbbbbb (3) ccccc (4) ddddd (5) rrrrr请补充完整代码
```
#include<iostream>
#include<cstring>
using namespace std;
class CSTRING {
private:
char *p;
public:
CSTRING() {p = NULL;}
// 重载输入运算符
friend istream& operator>>(istream& is, CSTRING& str) {
char tmp[100];
is >> tmp;
if (str.p != NULL) {
delete[] str.p;
}
str.p = new char[strlen(tmp) + 1];
strcpy(str.p, tmp);
return is;
}
// 重载比较运算符
friend bool operator>(CSTRING& str1, CSTRING& str2) {
return strcmp(str1.p, str2.p) > 0;
}
// 重载输出运算符
friend ostream& operator<<(ostream& os, CSTRING& str) {
os << str.p;
return os;
}
~CSTRING() {
if (p != NULL) {
delete[] p;
}
}
};
int main() {
int n,i,j;
cin >> n;
CSTRING t,*cs = new CSTRING[n];
for (i = 0; i < n; i++) {
cin >> cs[i];
}
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (cs[j] > cs[j + 1]) {
t = cs[j];
cs[j] = cs[j + 1];
cs[j + 1] = t;
}
}
}
for (i = 0; i < n; i++) {
cout << "(" << i + 1 << ") " << cs[i] << endl;
}
delete[] cs;
return 0;
}
```
<style name="SwitchThemeChat3D"> <item name="colorControlActivated">@color/White</item> <item name="colorSwitchThumbNormal">#5AAAF6</item> <item name="android:colorForeground">#BBBBBB</item> <item name="android:colorForegroundInverse">#FFFFFF</item> <item name="android:colorBackground">#FFFFFF</item> <item name="android:colorPrimary">#5AAAF6</item> <item name="android:thumbTint">#5AAAF6</item> </style>
这是一个样式定义,名为"SwitchThemeChat3D"。它定义了一些颜色属性,如开关按钮的背景颜色、前景颜色和滑块颜色等。其中,"colorControlActivated"是控制激活状态的颜色,"colorSwitchThumbNormal"是滑块的正常状态颜色,"android:colorForeground"是前景颜色,"android:colorForegroundInverse"是反向前景颜色,"android:colorBackground"是背景颜色,"android:colorPrimary"是主要颜色,"android:thumbTint"是滑块的颜色。这个样式可能是用于聊天应用程序中的开关按钮等控件的样式。
阅读全文
相关推荐
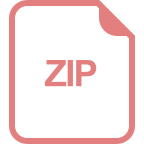
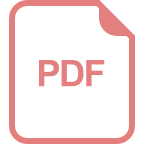
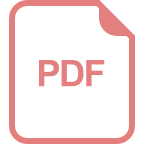
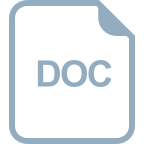
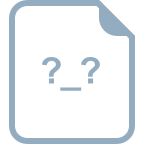
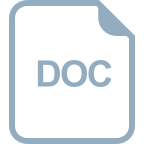
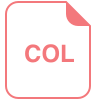







