C语言 jpg转RGB565 完整实现
时间: 2024-11-25 17:19:56 浏览: 50
完整的C语言实现将涉及多个库和步骤。这里提供一个简化版的示例,假设我们已经包含了必要的库(如jpeglib),并且有一个名为`write_rgb565_to_image`的函数用于保存转换后的RGB565像素到文件或内存。以下是基本的代码框架:
```c
#include <stdio.h>
#include <jpeglib.h>
typedef struct {
JOCTET* buffer; // JPEG解码后的临时缓冲区
JDIMENSION width; // 输出宽度
JDIMENSION height; // 输出高度
} MyDecompressor;
void init_jpeg_decompress(MyDecompressor* decoder, FILE* input)
{
jpeg_create_decompress(decoder);
jpeg_stdio_src(decoder->buffer, input);
jpeg_read_header(decoder, TRUE); // 读取头部信息
}
void process_image(MyDecompressor* decoder, void (*write_pixel)(unsigned short, int, int))
{
if (decoder->error_mgr.err == JERR_BOGUS_PARAM) {
printf("Invalid parameter.\n");
return;
}
jpeg_start_decompress(decoder);
// 创建RGB565数组
unsigned short* rgb565_buffer = malloc(decoder->output_width * decoder->output_height * sizeof(unsigned short));
while (jpeg_read_scanlines(decoder, decoder->buffer, 1) == OK) {
for (int y = 0; y < decoder->output_height; ++y) {
for (int x = 0; x < decoder->output_width; ++x) {
int pixel = decoder->buffer[x];
// RGB565转换
int b = (pixel >> 11) & 0x1F; // 5位蓝
int g = (pixel >> 5) & 0x3F; // 6位绿
int r = pixel & 0x1F; // 5位红
// 结合到16位RGB565格式
unsigned short rgb565 = ((r << 11) | (g << 5) | b) & 0xFFFF;
write_pixel(rgb565, x, y); // 调用用户提供的写像素函数
}
}
}
jpeg_finish_decompress(decoder);
free(rgb565_buffer);
}
void save_to_file(MyDecompressor* decoder, char* output_filename)
{
FILE* output = fopen(output_filename, "wb");
if (output == NULL) {
perror("Failed to open output file");
return;
}
for (int y = 0; y < decoder->height; ++y) {
for (int x = 0; x < decoder->width; ++x) {
unsigned short pixel = read_pixel_from_image(x, y); // 假设此处有获取已转换像素的函数
fwrite(&pixel, sizeof(pixel), 1, output);
}
}
fclose(output);
}
int main()
{
MyDecompressor decompressor;
init_jpeg_decompress(&decompressor, stdin); // 以标准输入作为源
// 处理图像
process_image(&decompressor, save_to_file);
return 0;
}
```
注意,这个示例假设了存在一些辅助函数,比如`jpeg_stdio_src`、`jpeg_start_decompress`等。实际项目中,你需要根据所使用的jpeg库文档来调整这些部分。
阅读全文
相关推荐
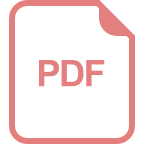
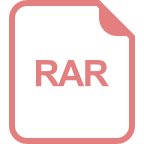
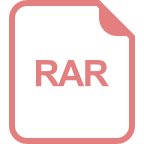

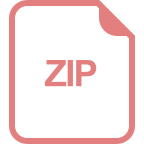

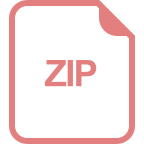
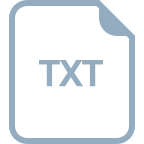
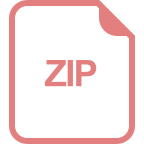
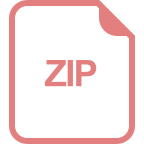
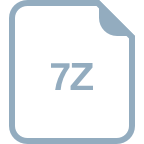
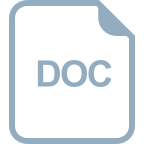
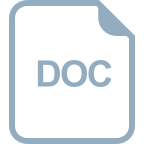
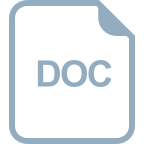
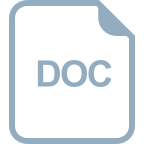
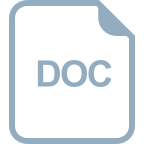
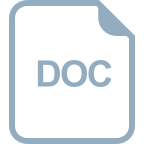
