implementation "androidx.compose.material3:material3:$material3_version"怎么用
时间: 2024-10-02 22:01:59 浏览: 79
`implementation "androidx.compose.material3:material3:$material3_version"` 是在Android项目中的Gradle构建脚本(build.gradle)中引入Material 3库的方式。`$material3_version` 是变量名,你需要替换成实际的Material 3版本号,例如 `1.4.0-alpha01`。
在`build.gradle`文件(通常在`app`模块下的`dependencies`部分)添加这一行,意思是当你设置了这个变量(例如在项目的`settings.gradle`或顶部级别的`build.gradle`中), Gradle会自动下载并关联指定版本的Material 3组件,以便在你的应用中使用Material Design 3的UI组件和样式。
举个例子:
```groovy
// 如果你想要使用1.4.0-alpha01版本
dependencies {
implementation "androidx.compose.material3:material3:1.4.0-alpha01"
}
```
然后运行`gradlew build`或`./gradlew build`命令来同步依赖并更新你的项目。
相关问题
plugins { id 'com.android.application' id 'org.jetbrains.kotlin.android' } android { namespace 'com.example.qrtopicture' compileSdk 33 defaultConfig { applicationId "com.example.qrtopicture" minSdk 24 targetSdk 33 versionCode 1 versionName "1.0" testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner" vectorDrawables { useSupportLibrary true } } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } compileOptions { sourceCompatibility JavaVersion.VERSION_1_8 targetCompatibility JavaVersion.VERSION_1_8 } kotlinOptions { jvmTarget = '1.8' } buildFeatures { compose true } composeOptions { kotlinCompilerExtensionVersion '1.3.2' } packagingOptions { resources { excludes += '/META-INF/{AL2.0,LGPL2.1}' } } } dependencies { implementation 'com.google.zxing:core:3.4.1' implementation 'com.google.zxing:android-core:3.3.0' implementation 'com.google.zxing:android-integration:3.3.0' implementation 'androidx.appcompat:appcompat:1.4.0' implementation 'androidx.core:core-ktx:1.8.0' implementation 'androidx.lifecycle:lifecycle-runtime-ktx:2.3.1' implementation 'androidx.activity:activity-compose:1.5.1' implementation platform('androidx.compose:compose-bom:2022.10.00') implementation 'androidx.compose.ui:ui' implementation 'androidx.compose.ui:ui-graphics' implementation 'androidx.compose.ui:ui-tooling-preview' implementation 'androidx.compose.material3:material3' testImplementation 'junit:junit:4.13.2' androidTestImplementation 'androidx.test.ext:junit:1.1.5' androidTestImplementation 'androidx.test.espresso:espresso-core:3.5.1' androidTestImplementation platform('androidx.compose:compose-bom:2022.10.00') androidTestImplementation 'androidx.compose.ui:ui-test-junit4' debugImplementation 'androidx.compose.ui:ui-tooling' debugImplementation 'androidx.compose.ui:ui-test-manifest' }帮我看看
你的 build.gradle 文件看起来没有问题,但是你可能需要检查一下你的项目中是否包含 `com.example.qrtopicture.MainActivity` 类文件,并且这个类文件是否有正确的命名空间。另外,你可能需要检查一下你的 AndroidManifest.xml 文件中是否正确声明了这个 Activity。如果还是无法解决问题,你可以尝试使用 Android Studio 自带的“Sync with Gradle Files”功能,或者尝试清除项目的构建缓存,重新构建项目。如果还是无法解决问题,可能需要进一步排查问题原因。
androidx compose
### AndroidX Compose 使用教程
#### 环境配置
为了开始使用 Jetpack Compose 进行开发,环境设置是必不可少的第一步。这涉及到更新 Gradle 插件到最新版本以及添加必要的依赖项来支持 Composable 函数的编写和预览功能[^1]。
```gradle
plugins {
id 'com.android.application' version '7.2.0' apply false
}
dependencies {
implementation "androidx.compose.ui:ui:$compose_version"
implementation "androidx.compose.material:material:$compose_version"
implementation "androidx.compose.ui:ui-tooling-preview:$compose_version"
}
```
#### 创建首个Composable函数
Jetpack Compose 中的核心概念之一就是 `@Composable` 函数,这些函数用于描述 UI 组件及其状态。下面是一个简单的例子展示如何定义一个可组合的小部件:
```kotlin
import androidx.compose.runtime.*
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.unit.sp
@Composable
fun Greeting(name: String) {
Text(text = "Hello $name!", style = TextStyle(fontSize = 24.sp))
}
```
#### 列表显示
当处理动态数据集时,可以利用 `LazyColumn` 和其他类似的布局组件轻松创建滚动列表。通过导入特定的扩展方法如 `items()` 可以简化遍历集合的过程,并高效地渲染每一项内容[^2]。
```kotlin
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.items
import androidx.compose.material.Text
val list = mutableListOf<String>().apply {
for (i in 0 until 100) add("Item >> ${i}")
}
@Composable
fun ItemList() {
LazyColumn {
items(list) { item ->
Text(text = "Item >> ${item}")
}
}
}
```
阅读全文
相关推荐















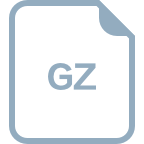
