Android开发 compose组件 数据库查询返回Flow<List<NoteFolderEntity>> list 正在使用LazyColumn显示 ,当item超过屏幕后,添加数据到第0位置时 没有动画显示,如何解决 要求写完整代码示例
时间: 2024-05-04 10:19:09 浏览: 10
好的,我理解你的要求。下面是一个简单的示例代码,演示如何使用Compose组件、Room数据库和Flow在Android应用中查询数据、添加数据,并通过动画显示添加的数据:
首先,在你的build.gradle文件中添加以下依赖项:
```groovy
dependencies {
// ...
// Compose
implementation "androidx.compose.ui:ui:$compose_version"
implementation "androidx.compose.material:material:$compose_version"
implementation "androidx.compose.runtime:runtime:$compose_version"
// Room
implementation "androidx.room:room-runtime:$room_version"
kapt "androidx.room:room-compiler:$room_version"
implementation "androidx.room:room-ktx:$room_version"
// Kotlin coroutines
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-core:$coroutines_version"
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-android:$coroutines_version"
}
```
其中 `$compose_version`、`$room_version` 和 `$coroutines_version` 是你使用的Compose、Room和Kotlin Coroutines版本号,你需要替换为你自己的版本号。
接下来,在你的应用中创建一个数据表格,并定义一个数据类,描述你存储在这个表格中的数据:
```kotlin
@Entity(tableName = "note_folder")
data class NoteFolderEntity(
@PrimaryKey(autoGenerate = true)
val id: Long = 0L,
val name: String,
val description: String = ""
)
```
然后,创建一个数据访问对象(DAO),定义用于获取、添加和删除数据的方法:
```kotlin
@Dao
interface NoteFolderDao {
@Query("SELECT * FROM note_folder")
fun getAll(): Flow<List<NoteFolderEntity>>
@Insert(onConflict = OnConflictStrategy.IGNORE)
suspend fun insertAll(vararg noteFolderEntities: NoteFolderEntity)
@Query("DELETE FROM note_folder WHERE id = :id")
suspend fun deleteById(id: Long)
}
```
在你的应用中创建一个Room数据库实例,并将数据表格和数据访问对象与之关联:
```kotlin
@Database(entities = [NoteFolderEntity::class], version = 1)
abstract class AppDatabase : RoomDatabase() {
abstract fun noteFolderDao(): NoteFolderDao
companion object {
const val DATABASE_NAME = "note_database"
@Volatile private var INSTANCE: AppDatabase? = null
fun getInstance(context: Context): AppDatabase {
synchronized(this) {
var instance = INSTANCE
if(instance == null) {
instance = Room.databaseBuilder(
context.applicationContext,
AppDatabase::class.java,
DATABASE_NAME
).build()
INSTANCE = instance
}
return instance
}
}
}
}
```
现在,你可以在你的Android应用的任何地方使用Room数据库查询数据和添加数据了。下面是一个使用Compose组件的界面示例,它可以显示从数据库中检索的数据,并显示添加到数据列表中的新数据。此外,当新数据添加时会播放一个简单的动画:
```kotlin
@Composable
fun NoteListScreen(db: AppDatabase) {
val noteFolderEntities = db.noteFolderDao().getAll().collectAsState(initial = emptyList())
// Track the currently visible notes
val visibleNotes = remember { mutableStateListOf(*noteFolderEntities.value.toTypedArray()) }
// Add a new note to the beginning of the list, with a name corresponding to the current time
val addNote = {
val time = System.currentTimeMillis()
val note = NoteFolderEntity(
name = "Note $time",
description = "Created at ${Date(time)}"
)
visibleNotes.add(0, note)
db.noteFolderDao().insertAll(note)
}
Scaffold(
topBar = { TopAppBar(title = { Text("Notes") }) }
) {
Column {
Button(onClick = addNote) {
Text("Add Note")
}
LazyColumn {
items(visibleNotes) { note ->
val backgroundColor = remember { mutableStateOf(Color.Transparent) }
// Animate the background color of the list item when it is added
val animateBackground = rememberInfiniteTransition()
val colorAnimation = animateBackground.color(
targetValue = Color.Yellow,
animationSpec = infiniteRepeatable(
animation = tween(2000),
repeatMode = RepeatMode.Reverse
)
)
Box(
Modifier
.background(colorAnimation.value)
.padding(8.dp)
) {
Text(note.name)
}
}
}
}
}
}
```
注意,这只是一个简单的示例代码,只查询单个表,并且不使用LiveData来检测数据变化。在实际的应用中,你可能会查询多个表,并使用LiveData来检测数据变化。此外,你可能需要使用更复杂的动画效果来显示添加到列表中的新数据。
相关推荐
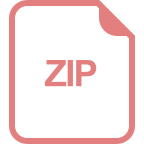








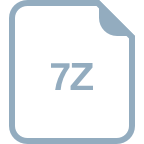
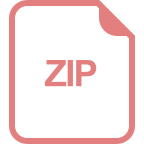
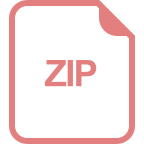
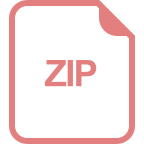
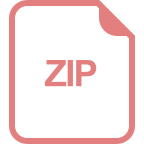
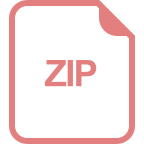
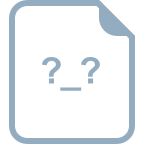