用c语言给定含有n个不同的数的数组L=<x,…,x,如果L中存在x,使得x<x<…x„<>xm>…>x„,则称L是单峰的,并称x,是L的“峰顶”,假设L是单峰的,设计一个算法找到L的峰顶。 要求:1.写出算法伪代码2.分析算法复杂度3.编写一个程序
时间: 2024-10-11 17:11:33 浏览: 21
算法伪代码:
```pseudo
function findPeak(L):
n = length of L
if n <= 1:
return null (因为只有一个元素或者空数组,不存在峰值)
left = 0
right = n - 1
peak_index = -1
while left < right:
mid = (left + right) / 2
if L[mid] < L[mid + 1]:
# 如果中间元素小于右侧元素,那么峰顶可能在右半部分
left = mid + 1
else:
# 如果中间元素大于等于右侧元素,那么峰顶可能在左半部分或中间
right = mid
# 左侧边界就是峰顶位置,因为在二分查找过程中,已确定左侧都是非峰值
peak_index = left
if peak_index != -1 and L[peak_index] < L[peak_index + 1]: # 检查是否真的有峰值
return peak_index
else:
return null # 如果不是峰值,则返回null
end function
```
算法复杂度分析:
- 时间复杂度:该算法采用了二分查找法,最坏情况下需要比较log(n)次,所以时间复杂度为O(log n)。
- 空间复杂度:算法只需要常数级别的额外空间用于存储变量,所以空间复杂度为O(1)。
C语言程序实现:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_SIZE 100
bool isPeak(int arr[], int size, int index) {
if (index == 0 || index == size - 1)
return true;
if (arr[index] < arr[index + 1])
return false;
return isPeak(arr, size, index + 1);
}
int findPeakIndex(int arr[], int size) {
int left = 0;
int right = size - 1;
while (left < right) {
int mid = (left + right) / 2;
if (!isPeak(arr, size, mid)) {
left = mid + 1;
} else {
right = mid;
}
}
if (isPeak(arr, size, left))
return left;
return -1;
}
int main() {
int arr[MAX_SIZE];
int n, i, peakIndex;
printf("Enter the number of elements in the array: ");
scanf("%d", &n);
printf("Enter the array elements: ");
for (i = 0; i < n; ++i) {
scanf("%d", &arr[i]);
}
peakIndex = findPeakIndex(arr, n);
if (peakIndex != -1)
printf("The peak element is at index %d with value %d.\n", peakIndex, arr[peakIndex]);
else
printf("No peak found in the given array.\n");
return 0;
}
```
这个程序会首先读取数组长度和元素,然后调用`findPeakIndex`函数来找出峰顶的位置。如果找不到峰顶,就提示没有峰。注意这里假设输入的数组大小不超过MAX_SIZE。
阅读全文
相关推荐
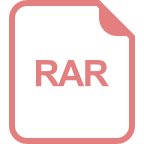
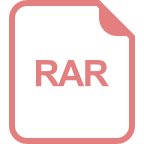
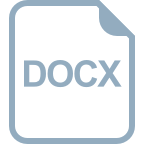
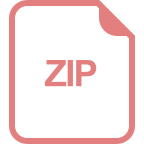
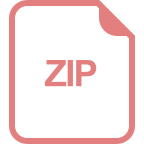
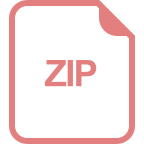
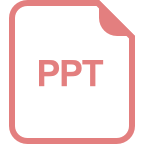
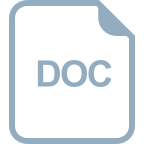
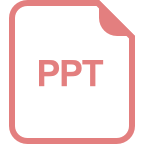
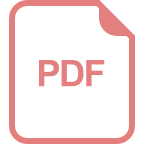
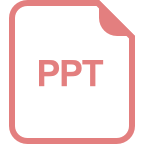
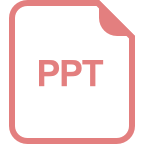
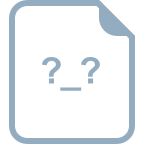
