C#编写一段批量读100个int属性和批量写100个int属性的代码,其中属性名分别为MyProperty1~MyProperty100。要求:为了提高性能使用Action委托的方式读写属性,禁止使用GetValue和SetValue来读写属性
时间: 2024-10-20 15:07:03 浏览: 21
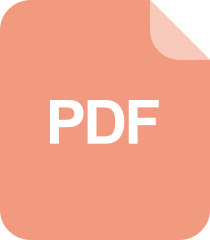
深入理解C#索引器(一种支持参数的属性)与属性的对比
在C#中,我们可以利用反射和Action委托来批量读取和写入具有特定名称的整型属性,避免直接使用`GetValue`和`SetValue`。以下是一个示例:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
public class MyClass
{
[System.Runtime.Serialization.DataMember]
public int MyProperty1 { get; set; }
// ... 其他99个MyPropertyi...
// Action委托用于读写属性值
private static readonly Action<MyClass, string, int> ReadWriteProperty = (obj, propertyName, value) =>
{
PropertyInfo propertyInfo = obj.GetType().GetProperty(propertyName);
if (propertyInfo != null)
propertyInfo.SetValue(obj, value, null); // 写操作
else
Console.WriteLine($"无法找到属性'{propertyName}'");
};
public void BatchReadWrite()
{
List<string> propertyNames = new List<string> { "MyProperty1", /*...*/ "MyProperty100" };
Random random = new Random();
for (int i = 1; i <= 100; i++)
{
MyClass obj = new MyClass(); // 创建对象实例
// 批量读取100个属性值
foreach (string propertyName in propertyNames)
Console.WriteLine($"Property {propertyName}: {ReadWriteProperty(obj, propertyName, i)}");
// 批量随机写入100个新值
int newValue = random.Next(int.MinValue, int.MaxValue);
foreach (string propertyName in propertyNames)
ReadWriteProperty(obj, propertyName, newValue);
}
}
}
// 调用方法
MyClass myObject = new MyClass();
myObject.BatchReadWrite();
```
在这个例子中,我们创建了一个名为`ReadWriteProperty`的动作委托,它接受一个对象、属性名称和一个值作为输入,并尝试通过反射设置或获取该属性。然后在`BatchReadWrite`方法中,我们遍历每个属性名称并执行对应的读写操作。
阅读全文
相关推荐
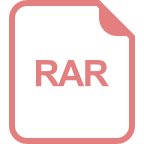
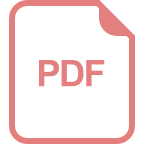

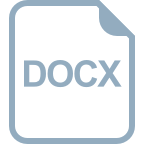
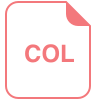
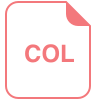
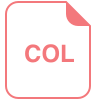
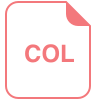









