python爬虫爬取新浪新闻
时间: 2023-10-03 18:06:01 浏览: 294
你可以使用 Python 中的一些库来编写爬虫程序,例如 requests 和 BeautifulSoup。下面是一个简单的示例,展示如何使用这些库来爬取新浪新闻:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://news.sina.com.cn/'
response = requests.get(url)
html = response.content
soup = BeautifulSoup(html, 'html.parser')
news_list = soup.find_all('a')
for news in news_list:
print(news.text)
```
这段代码首先使用 `requests` 库发送一个 GET 请求获取网页内容,然后用 `BeautifulSoup` 解析 HTML。你可以根据需要使用各种方法来提取你想要的数据。在上面的示例中,我们通过查找所有的 `<a>` 标签来获取新闻标题。你可以根据具体的网页结构进行适当的调整。
相关问题
python爬虫爬取新浪新闻标题
可以使用Python的requests和BeautifulSoup库来编写爬虫代码,以获取新浪新闻的标题。首先,需要安装requests和BeautifulSoup4库。打开命令提示符并输入以下命令:
```
pip install requests
pip install BeautifulSoup4
```
安装完成后,可以按照以下步骤编写爬虫代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://news.sina.com.cn/china/'
res = requests.get(url)
res.encoding = 'UTF-8'
soup = BeautifulSoup(res.text, 'html.parser')
for news in soup.select('.news-item'):
h2 = news.select('h2')
if len(h2) > 0:
title = h2[0].text
print(title)
```
这段代码将爬取新浪新闻页面上所有新闻的标题,并将其打印出来。
请用Python爬虫爬取新浪新闻,要求有新闻类型,正文内容,标题,作者,发布时间,点赞数,输出类型为json
好的,以下是Python爬虫爬取新浪新闻并输出json格式数据的示例代码:
```python
import requests
from bs4 import BeautifulSoup
import json
# 设置请求头,模拟浏览器访问
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
# 新浪新闻首页
url = 'https://news.sina.com.cn/'
# 发送get请求
response = requests.get(url, headers=headers)
# 解析html
soup = BeautifulSoup(response.text, 'lxml')
# 获取新闻列表
news_list = soup.select('.news-2')
# 遍历新闻列表,提取数据
data_list = []
for news in news_list:
# 新闻类型
news_type = news.select('.news-type')[0].text
# 标题
title = news.select('.news-title')[0].text
# 链接
link = news.select('.news-title')[0].get('href')
# 作者
author = news.select('.news-source')[0].text.strip()
# 发布时间
pub_time = news.select('.news-time')[0].text.strip()
# 点赞数
like_num = news.select('.num')[0].text
# 正文内容
resp = requests.get(link, headers=headers)
content_soup = BeautifulSoup(resp.text, 'lxml')
content = content_soup.select('.article')[0].text.strip()
# 构造数据字典
data = {'news_type': news_type,
'title': title,
'link': link,
'author': author,
'pub_time': pub_time,
'like_num': like_num,
'content': content}
# 将数据字典添加到列表中
data_list.append(data)
# 输出json格式数据
json_data = json.dumps(data_list, ensure_ascii=False)
print(json_data)
```
以上代码将爬取新浪新闻首页的新闻数据,并输出json格式数据,包括新闻类型、正文内容、标题、作者、发布时间、点赞数等信息。
阅读全文
相关推荐
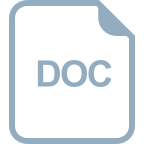
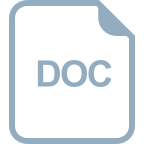
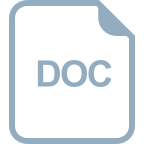
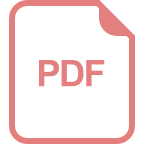





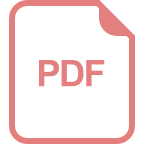
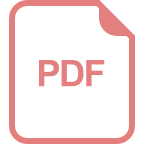


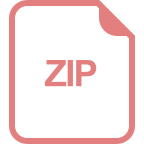
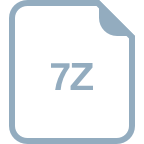