python多线程启动与停止
时间: 2024-11-03 07:22:27 浏览: 25
在Python中,多线程通常使用内置的`threading`模块来实现。启动和停止一个线程的基本步骤如下:
**启动线程:**
```python
import threading
def worker_function():
# 线程要执行的任务
print('Worker thread is running...')
# 创建一个新的线程对象
worker_thread = threading.Thread(target=worker_function)
# 启动线程
worker_thread.start()
```
在这里,`worker_function`是一个要在新线程中运行的函数,`Thread`类的实例`worker_thread`则是线程的代表,`start()`方法用于实际启动线程。
**停止线程:**
Python的`Thread`对象并没有提供直接停止线程的方法,因为线程一旦开始,除非它自然结束(例如,函数返回或遇到`sys.exit()`),否则无法强制停止。如果你想要让线程提前结束,一种常见的做法是在需要停止的地方设置一个标志,然后在线程内部检查这个标志。
例如,在`worker_function`中添加一个全局变量`stop_event`:
```python
import threading
import time
STOP_EVENT = threading.Event()
def worker_function(stop_event):
while not stop_event.is_set(): # 检查事件是否设置
print('Working...')
time.sleep(1) # 假设这是耗时任务
if stop_event.wait(1): # 如果设置了事件,就立即退出循环
print('Thread stopped by user.')
# 同样创建线程实例
worker_thread = threading.Thread(target=worker_function, args=(STOP_EVENT,))
worker_thread.start()
```
如果你想停止线程,只需调用`stop_event.set()`即可。
注意:由于线程的同步问题,这种做法可能不是最推荐的方式,特别是在并发环境下。更好的解决方案通常是使用`threading.Event`配合`queue.Queue`来进行线程间通信。
阅读全文
相关推荐
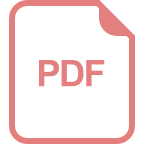
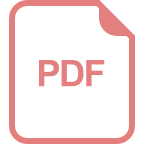
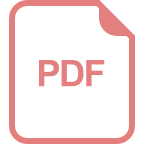
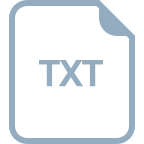
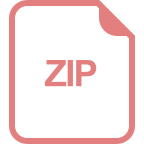
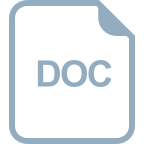
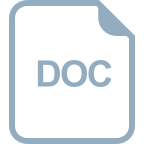
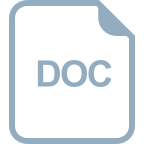
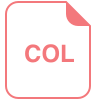








