如何在php进行序列化传输到Python进行反序列化再返回php
时间: 2024-10-18 20:11:16 浏览: 16
在PHP中,你可以使用`json_encode`函数将数据序列化成JSON格式,然后通过HTTP请求发送到Python应用。Python应用收到后,可以使用`json.loads`函数反序列化数据。以下是简单的步骤:
**在PHP中序列化并发送到Python:**
```php
$data = array('name' => 'John', 'age' => 30);
$json_data = json_encode($data); // 序列化数据
// 发送HTTP请求到Python端
$url = "http://your-python-api.com/receive";
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $json_data);
$response = curl_exec($ch);
curl_close($ch);
// Python会返回响应
```
**在Python中接收并反序列化:**
```python
import json
# 定义接收函数
def receive_request(data):
deserialized_data = json.loads(data) # 反序列化
return deserialized_data
# 假设从HTTP请求获取了数据
received_data = receive_request(json_data)
```
**在Python中处理完数据后返回给PHP:**
Python处理完数据后,同样可以将其序列化为JSON格式,并设置HTTP响应头为application/json,然后返回给PHP。这里假设Python有个名为`send_response`的函数:
```python
response_data = {'message': 'Processed data'} # 示例数据
response_json = json.dumps(response_data)
# 假设通过Flask等框架设置HTTP响应
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/return', methods=['POST'])
def return_data():
response = jsonify(response_data)
response.headers['Content-Type'] = 'application/json'
return response
```
**再次在PHP中接收并处理:**
```php
$received_response = file_get_contents($response_body); // 获取返回的HTTP响应
$decoded_response = json_decode($received_response, true); // 解析JSON
// 现在可以处理$decoded_response
```
阅读全文
相关推荐
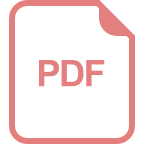
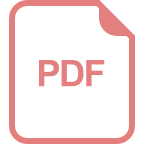
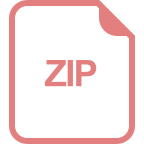
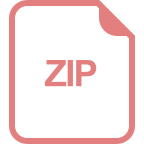
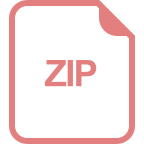
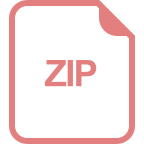
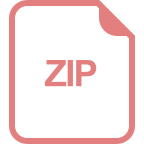
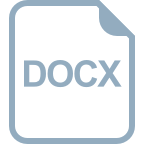
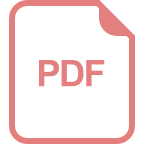
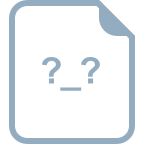
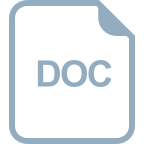
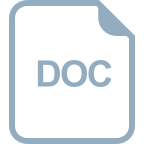
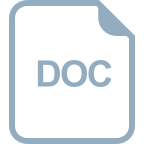
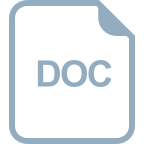
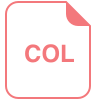
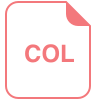
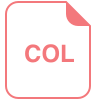
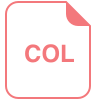