if else elif
时间: 2024-08-12 08:02:23 浏览: 40
`if...else` 和 `elif`(即 `else if`)是常用的条件控制结构,在许多编程语言中,包括Python、JavaScript等都支持。它们用于程序流程控制,根据特定条件执行相应的代码块。
当你有一个需要检查多个条件的情况时,可以使用这个结构。基本语法如下:
```python
if condition1:
# 如果 condition1 为真,则执行这里的代码
else:
# 否则(如果 condition1 为假),执行这里
elif condition2: # 可选的部分,如果 condition1 已经为假
# 如果 condition2 为真,则执行这里的代码,不会继续检查后面的条件
else:
# 如果所有之前的条件都不满足,执行这里的代码
```
`elif` 用于增加额外的条件判断,只有当前面的条件都不成立时,才会检查这个条件。这种结构使得程序能够根据给定的顺序检查一系列可能的条件,提高代码的灵活性。
相关问题
if else elif
If-else and elif are conditional statements in programming languages that allow the program to execute different sets of instructions based on certain conditions.
If statement: It is a basic conditional statement that executes a set of instructions if a condition is true. If the condition is false, the program will skip the instructions in the if block.
Syntax:
if condition:
#execute instructions if condition is true
Example:
x = 10
if x > 5:
print("x is greater than 5")
Output: x is greater than 5
Else statement: It is used after an if statement to execute a set of instructions when the condition is false.
Syntax:
if condition:
#execute instructions if condition is true
else:
#execute instructions if condition is false
Example:
x = 3
if x > 5:
print("x is greater than 5")
else:
print("x is less than or equal to 5")
Output: x is less than or equal to 5
Elif statement: It is used to check additional conditions after the if statement. It allows the program to execute different sets of instructions based on multiple conditions.
Syntax:
if condition1:
#execute instructions if condition1 is true
elif condition2:
#execute instructions if condition2 is true
else:
#execute instructions if both condition1 and condition2 are false
Example:
x = 7
if x > 10:
print("x is greater than 10")
elif x > 5:
print("x is greater than 5 but less than or equal to 10")
else:
print("x is less than or equal to 5")
Output: x is greater than 5 but less than or equal to 10
if else elif else
if else elif else是一种在编程中用于控制程序流程的语句结构。它可以根据条件的不同执行不同的代码块。下面是对if else elif else的介绍:
1. if语句:if语句用于判断一个条件是否为真,如果条件为真,则执行if语句块中的代码。如果条件为假,则跳过if语句块,继续执行后续的代码。
2. else语句:else语句紧跟在if语句之后,用于处理if条件为假的情况。如果if条件为假,则执行else语句块中的代码。
3. elif语句:elif语句是if语句的扩展,用于判断多个条件。如果前面的if条件为假,可以使用elif语句来判断下一个条件是否为真。如果elif条件为真,则执行elif语句块中的代码。
4. else语句:else语句是在所有if和elif条件都为假时执行的代码块。它是可选的,可以省略。
下面是一个示例代码,演示了if else elif else的用法:
```
num = 10
if num > 0:
print("num是正数")
elif num < 0:
print("num是负数")
else:
print("num是零")
```
在这个示例中,首先判断num是否大于0,如果是,则输出"num是正数";如果不是,则判断num是否小于0,如果是,则输出"num是负数";如果前面的条件都不满足,则执行else语句块,输出"num是零"。
阅读全文
相关推荐
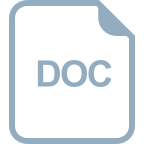
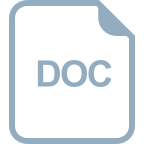
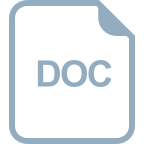













