编写一个Java程序,用二分查找法去查找含有10个元素的数组中某一个数据。
时间: 2024-11-13 15:27:51 浏览: 28
在Java中,你可以编写如下的程序来使用二分查找法(Binary Search)在一个有序数组中查找特定的数据。假设我们有一个整数数组arr[],我们可以创建一个名为binarySearch的方法:
```java
public class BinarySearchExample {
int binarySearch(int arr[], int x) {
int low = 0;
int high = arr.length - 1;
// 当数组长度为零或者我们要查找的元素小于最小值或大于最大值时,返回 -1
if (high < 0 || x > arr[high] || x < arr[low]) {
return -1;
}
while (low <= high) {
int mid = low + (high - low) / 2; // 计算中间索引
// 如果中间元素就是要找的,返回其索引
if (arr[mid] == x)
return mid;
// 如果中间元素比目标大,则在左半部分继续搜索
else if (arr[mid] > x)
high = mid - 1;
// 否则在右半部分搜索
else
low = mid + 1;
}
// 没有找到,返回 -1
return -1;
}
// 测试函数
public static void main(String args[]) {
BinarySearchExample bs = new BinarySearchExample();
int arr[] = {2, 3, 4, 10, 40, 50, 60, 70, 80, 90};
int n = arr.length;
int x = 50;
int result = bs.binarySearch(arr, x);
if (result == -1)
System.out.println("Element not present in array");
else
System.out.println("Element found at index " + result);
}
}
```
在这个例子中,`binarySearch`方法接收一个已排序的数组和要查找的元素x。它会反复将搜索范围减半,直到找到x或者搜索范围为空。如果找不到x,就返回-1。
阅读全文
相关推荐
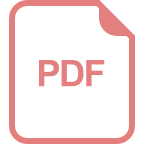
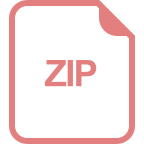
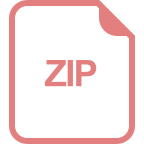
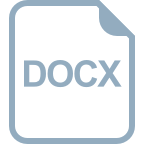
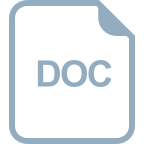







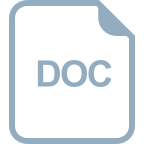





